# Basics
The MoonShine admin panel has an authentication system. By default it is enabled
but if you need to allow access for all users,
then it can be disabled in the configuration file config/moonshine.php
.
return [ // ... 'auth' => [ 'enable' => true, // ... ], // ...];
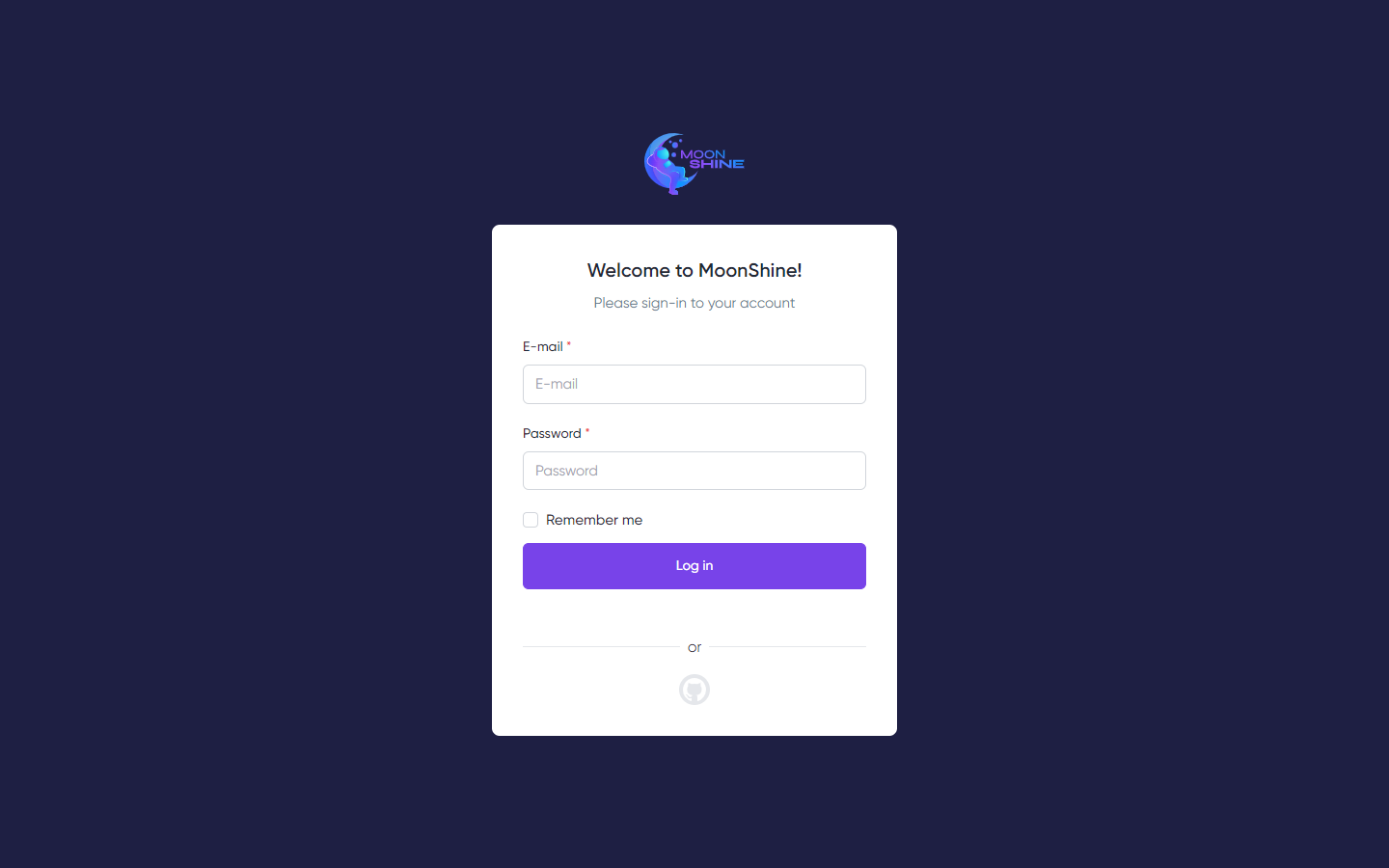
# Empowerment
If you use your own guard, provider, then they can be overridden in the configuration,
as well as the MoonshineUser
model.
return [ // ... 'auth' => [ // ... 'middleware' => Authenticate::class, 'guard' => 'moonshine', 'guards' => [ 'moonshine' => [ 'driver' => 'session', 'provider' => 'moonshine', ], ], 'providers' => [ 'moonshine' => [ 'driver' => 'eloquent', 'model' => MoonshineUser::class, ], ], // ... ], // ...];
# Login form
You can completely replace the login form with your own, just replace the class in the config with yours, and inside implement FormBuilder
return [ // ... 'forms' => [ 'login' => LoginForm::class ], // ...];
# Profile
You can completely replace the profile page with your own, just replace the page class in the config with yours
return [ // ... 'pages' => [ // ... 'profile' => ProfilePage::class ], // ...];
You can override profile fields in the configuration file config/moonshine.php
.
return [ // ... 'auth' => [ 'enable' => true, 'fields' => [ 'username' => 'email', 'password' => 'password', 'name' => 'name', 'avatar' => 'avatar' ], 'guard' => 'moonshine', // ... ], // ...];
If you don't want to use an avatar,
then specify 'avatar'=>''
or 'avatar'=>false
.
It is possible to change Guard in the Profile component.
Profile::make(guard: 'custom')
MoonShineAuth::guard('custom')->user()
# Pipelines
In the MoonShine admin panel it is possible to add logic to the authentication process, which will allow you to change the request or response object in the process.
To do this, you need to specify your Pipelines in the configuration file config/moonshine.php
.
return [ 'auth' => [ 'pipelines' => [ PipelineClass::class ], ]];
or
return [ 'auth' => [ 'pipelines' => [ new class { public function handle($request, $next) { return $next($request); } } ], ]];