The Card component allows you to create element cards.
You can create a Card using the static method make()
class Card
.
make(
Closure|string $title = '',
Closure|string|array $thumbnail = '',
Closure|string $url = '#',
Closure|array $values = [],
Closure|string|null $subtitle = null
)
make(
Closure|string $title = '',
Closure|string|array $thumbnail = '',
Closure|string $url = '#',
Closure|array $values = [],
Closure|string|null $subtitle = null
)
make(
Closure|string $title = '',
Closure|string|array $thumbnail = '',
Closure|string $url = '#',
Closure|array $values = [],
Closure|string|null $subtitle = null
)
make(
Closure|string $title = '',
Closure|string|array $thumbnail = '',
Closure|string $url = '#',
Closure|array $values = [],
Closure|string|null $subtitle = null
)
make(
Closure|string $title = '',
Closure|string|array $thumbnail = '',
Closure|string $url = '#',
Closure|array $values = [],
Closure|string|null $subtitle = null
)
$title
- card title,
$thumbnail
- images,
$url
- link,
$values
- list of values
$subtitle
- subtitle.
use MoonShine\Components\Card;
public function components(): array
{
return [
Card::make(
title: fake()->sentence(3),
thumbnail: '/images/image_1.jpg',
url: fn() => 'https://cutcode.dev',
values: ['ID' => 1, 'Author' => fake()->name()],
subtitle: date('d.m.Y')
)
];
}
use MoonShine\Components\Card;
//...
public function components(): array
{
return [
Card::make(
title: fake()->sentence(3),
thumbnail: '/images/image_1.jpg',
url: fn() => 'https://cutcode.dev',
values: ['ID' => 1, 'Author' => fake()->name()],
subtitle: date('d.m.Y')
)
];
}
//...
use MoonShine\Components\Card;
//...
public function components(): array
{
return [
Card::make(
title: fake()->sentence(3),
thumbnail: '/images/image_1.jpg',
url: fn() => 'https://cutcode.dev',
values: ['ID' => 1, 'Author' => fake()->name()],
subtitle: date('d.m.Y')
)
];
}
//...
use MoonShine\Components\Card;
//...
public function components(): array
{
return [
Card::make(
title: fake()->sentence(3),
thumbnail: '/images/image_1.jpg',
url: fn() => 'https://cutcode.dev',
values: ['ID' => 1, 'Author' => fake()->name()],
subtitle: date('d.m.Y')
)
];
}
//...
use MoonShine\Components\Card;
//...
public function components(): array
{
return [
Card::make(
title: fake()->sentence(3),
thumbnail: '/images/image_1.jpg',
url: fn() => 'https://cutcode.dev',
values: ['ID' => 1, 'Author' => fake()->name()],
subtitle: date('d.m.Y')
)
];
}
//...
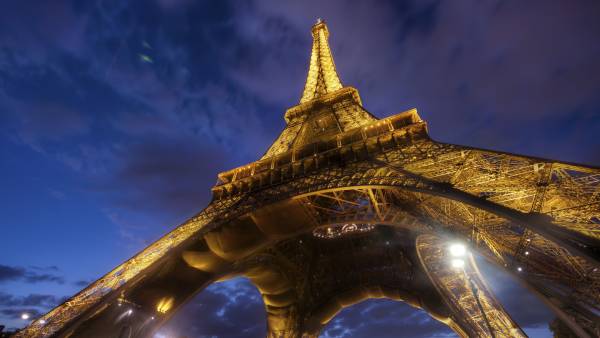
The header()
method allows you to set the header for cards.
header(Closure|string $value)
header(Closure|string $value)
header(Closure|string $value)
header(Closure|string $value)
header(Closure|string $value)
$value
- column or closure returning html code.
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_2.jpg',
)
->header(static fn() => Badge::make('new', 'success'))
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_2.jpg',
)
->header(static fn() => Badge::make('new', 'success'))
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_2.jpg',
)
->header(static fn() => Badge::make('new', 'success'))
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_2.jpg',
)
->header(static fn() => Badge::make('new', 'success'))
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_2.jpg',
)
->header(static fn() => Badge::make('new', 'success'))
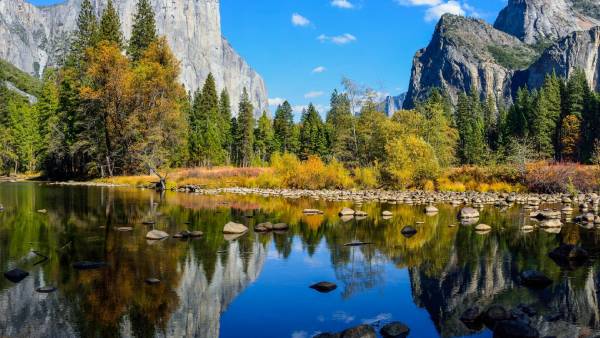
To add buttons to a card, you can use the actions()
method.
actions(Closure|string $value)
actions(Closure|string $value)
actions(Closure|string $value)
actions(Closure|string $value)
actions(Closure|string $value)
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_1.jpg',
)
->actions(
static fn() => ActionButton::make('Edit', route('name.edit'))
)
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_1.jpg',
)
->actions(
static fn() => ActionButton::make('Edit', route('name.edit'))
)
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_1.jpg',
)
->actions(
static fn() => ActionButton::make('Edit', route('name.edit'))
)
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_1.jpg',
)
->actions(
static fn() => ActionButton::make('Edit', route('name.edit'))
)
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_1.jpg',
)
->actions(
static fn() => ActionButton::make('Edit', route('name.edit'))
)
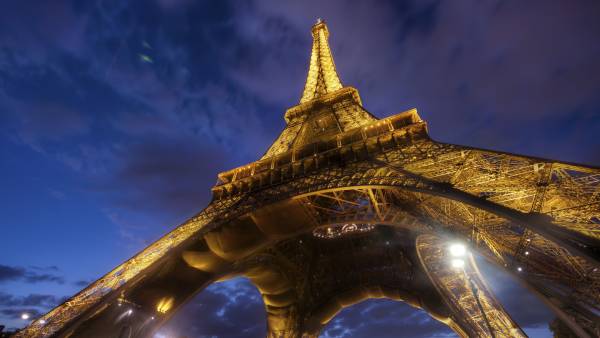
subtitle(Closure|string $value)
subtitle(Closure|string $value)
subtitle(Closure|string $value)
subtitle(Closure|string $value)
subtitle(Closure|string $value)
$value
- column or a closure that returns a subtitle.
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_2.jpg',
)
->subtitle(static fn() => 'Subtitle')
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_2.jpg',
)
->subtitle(static fn() => 'Subtitle')
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_2.jpg',
)
->subtitle(static fn() => 'Subtitle')
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_2.jpg',
)
->subtitle(static fn() => 'Subtitle')
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_2.jpg',
)
->subtitle(static fn() => 'Subtitle')
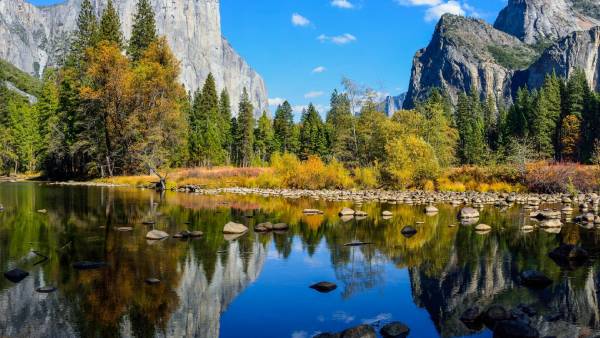
The url()
method allows you to set a link for the card title.
url(Closure|string $value)
url(Closure|string $value)
url(Closure|string $value)
url(Closure|string $value)
url(Closure|string $value)
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_1.jpg',
)
->url(static fn() => 'https://cutcode.dev')
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_1.jpg',
)
->url(static fn() => 'https://cutcode.dev')
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_1.jpg',
)
->url(static fn() => 'https://cutcode.dev')
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_1.jpg',
)
->url(static fn() => 'https://cutcode.dev')
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_1.jpg',
)
->url(static fn() => 'https://cutcode.dev')
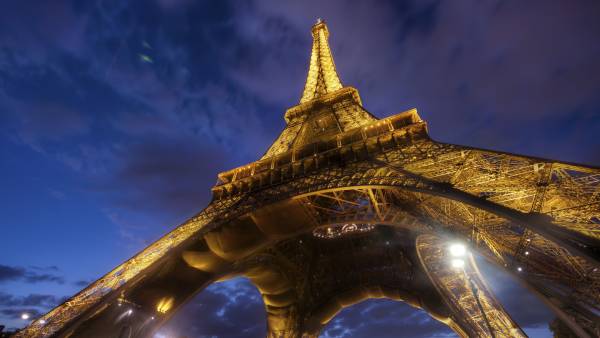
To add an images carousel to a card, you can use the thumbnails()
method.
thumbnails(Closure|string|array $value)
thumbnails(Closure|string|array $value)
thumbnails(Closure|string|array $value)
thumbnails(Closure|string|array $value)
thumbnails(Closure|string|array $value)
Cards::make(
title: fake()->sentence(3),
)
->thumbnail(['/images/image_2.jpg','/images/image_1.jpg'])
Cards::make(
title: fake()->sentence(3),
)
->thumbnail(['/images/image_2.jpg','/images/image_1.jpg'])
Cards::make(
title: fake()->sentence(3),
)
->thumbnail(['/images/image_2.jpg','/images/image_1.jpg'])
Cards::make(
title: fake()->sentence(3),
)
->thumbnail(['/images/image_2.jpg','/images/image_1.jpg'])
Cards::make(
title: fake()->sentence(3),
)
->thumbnail(['/images/image_2.jpg','/images/image_1.jpg'])
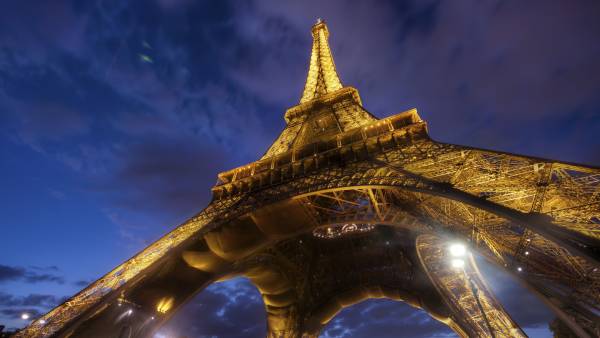
To add a list of values to a card, you can use the values()
method.
values(Closure|array $value)
values(Closure|array $value)
values(Closure|array $value)
values(Closure|array $value)
values(Closure|array $value)
$value
- associative array of values or closure.
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_1.jpg',
)
->values([
'ID' => 1,
'Author' => fake()->name()
])
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_1.jpg',
)
->values([
'ID' => 1,
'Author' => fake()->name()
])
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_1.jpg',
)
->values([
'ID' => 1,
'Author' => fake()->name()
])
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_1.jpg',
)
->values([
'ID' => 1,
'Author' => fake()->name()
])
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_1.jpg',
)
->values([
'ID' => 1,
'Author' => fake()->name()
])
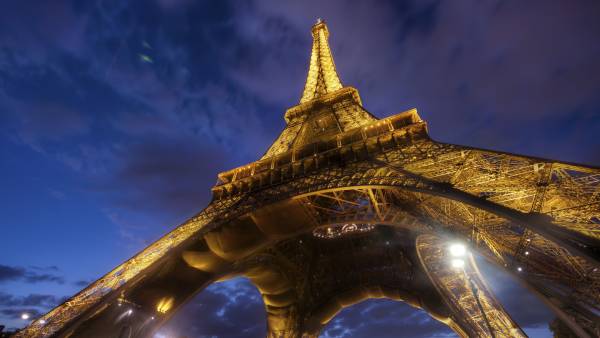
The overlay mode allows you to place the header and headings on top of the card image. This mode is activated by the overlay()
method of the same name.
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_2.jpg',
url: fn() => 'https://cutcode.dev',
values: ['ID' => 1, 'Author' => fake()->name()],
subtitle: date('d.m.Y')
)
->header(static fn() => Badge::make('new', 'success'))
->overlay()
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_2.jpg',
url: fn() => 'https://cutcode.dev',
values: ['ID' => 1, 'Author' => fake()->name()],
subtitle: date('d.m.Y')
)
->header(static fn() => Badge::make('new', 'success'))
->overlay()
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_2.jpg',
url: fn() => 'https://cutcode.dev',
values: ['ID' => 1, 'Author' => fake()->name()],
subtitle: date('d.m.Y')
)
->header(static fn() => Badge::make('new', 'success'))
->overlay()
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_2.jpg',
url: fn() => 'https://cutcode.dev',
values: ['ID' => 1, 'Author' => fake()->name()],
subtitle: date('d.m.Y')
)
->header(static fn() => Badge::make('new', 'success'))
->overlay()
Cards::make(
title: fake()->sentence(3),
thumbnail: '/images/image_2.jpg',
url: fn() => 'https://cutcode.dev',
values: ['ID' => 1, 'Author' => fake()->name()],
subtitle: date('d.m.Y')
)
->header(static fn() => Badge::make('new', 'success'))
->overlay()
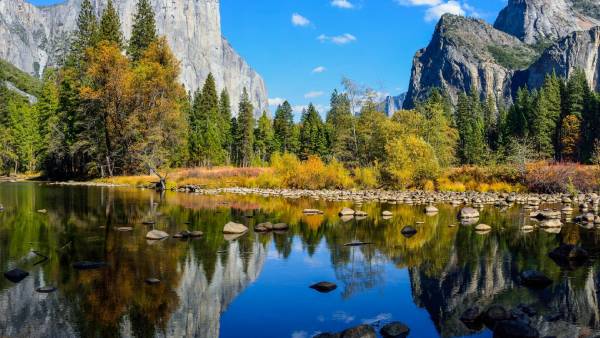