The Json field includes all the base methods.
Json has several methods to set the field structure:
keyValue()
, onlyValue()
and fields()
.
In the database, the field must be of text or json type. Also cast eloquent of an array or json or collection model.
The easiest way to work with a Json field is to use the keyValue()
method.
The result will be a simple json {key: value}
.
keyValue(
string $key = 'Key',
string $value = 'Value'
)
keyValue(
string $key = 'Key',
string $value = 'Value'
)
keyValue(
string $key = 'Key',
string $value = 'Value'
)
keyValue(
string $key = 'Key',
string $value = 'Value'
)
keyValue(
string $key = 'Key',
string $value = 'Value'
)
use MoonShine\Fields\Json;
public function fields(): array
{
return [
Json::make('Data')
->keyValue()
];
}
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->keyValue()
];
}
//...
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->keyValue()
];
}
//...
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->keyValue()
];
}
//...
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->keyValue()
];
}
//...
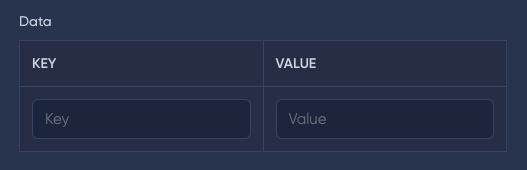
The default keys and values are the Text field, but you can use other fields for primitive data.
use MoonShine\Fields\Json;
use MoonShine\Fields\Select;
public function fields(): array
{
return [
Json::make('Label', 'data')->keyValue(
keyField: Select::make('Key')->options(['vk' => 'VK', 'email' => 'E-mail']),
valueField: Select::make('Value')->options(['1' => '1', '2' => '2']),
),
];
}
use MoonShine\Fields\Json;
use MoonShine\Fields\Select;
//...
public function fields(): array
{
return [
Json::make('Label', 'data')->keyValue(
keyField: Select::make('Key')->options(['vk' => 'VK', 'email' => 'E-mail']),
valueField: Select::make('Value')->options(['1' => '1', '2' => '2']),
),
];
}
//...
use MoonShine\Fields\Json;
use MoonShine\Fields\Select;
//...
public function fields(): array
{
return [
Json::make('Label', 'data')->keyValue(
keyField: Select::make('Key')->options(['vk' => 'VK', 'email' => 'E-mail']),
valueField: Select::make('Value')->options(['1' => '1', '2' => '2']),
),
];
}
//...
use MoonShine\Fields\Json;
use MoonShine\Fields\Select;
//...
public function fields(): array
{
return [
Json::make('Label', 'data')->keyValue(
keyField: Select::make('Key')->options(['vk' => 'VK', 'email' => 'E-mail']),
valueField: Select::make('Value')->options(['1' => '1', '2' => '2']),
),
];
}
//...
use MoonShine\Fields\Json;
use MoonShine\Fields\Select;
//...
public function fields(): array
{
return [
Json::make('Label', 'data')->keyValue(
keyField: Select::make('Key')->options(['vk' => 'VK', 'email' => 'E-mail']),
valueField: Select::make('Value')->options(['1' => '1', '2' => '2']),
),
];
}
//...
For more advanced use, use the fields()
method and pass the required set of fields.
As a result, the following json will be generated:
[{title: 'title', value: 'value', active: 'active'}]
fields(Fields|Closure|array $fields)
fields(Fields|Closure|array $fields)
fields(Fields|Closure|array $fields)
fields(Fields|Closure|array $fields)
fields(Fields|Closure|array $fields)
use MoonShine\Fields\Json;
use MoonShine\Fields\Position;
use MoonShine\Fields\Switcher;
use MoonShine\Fields\Text;
public function fields(): array
{
return [
Json::make('Product Options', 'options')
->fields([
Position::make(),
Text::make('Title'),
Text::make('Value'),
Switcher::make('Active')
])
];
}
use MoonShine\Fields\Json;
use MoonShine\Fields\Position;
use MoonShine\Fields\Switcher;
use MoonShine\Fields\Text;
//...
public function fields(): array
{
return [
Json::make('Product Options', 'options')
->fields([
Position::make(),
Text::make('Title'),
Text::make('Value'),
Switcher::make('Active')
])
];
}
//...
use MoonShine\Fields\Json;
use MoonShine\Fields\Position;
use MoonShine\Fields\Switcher;
use MoonShine\Fields\Text;
//...
public function fields(): array
{
return [
Json::make('Product Options', 'options')
->fields([
Position::make(),
Text::make('Title'),
Text::make('Value'),
Switcher::make('Active')
])
];
}
//...
use MoonShine\Fields\Json;
use MoonShine\Fields\Position;
use MoonShine\Fields\Switcher;
use MoonShine\Fields\Text;
//...
public function fields(): array
{
return [
Json::make('Product Options', 'options')
->fields([
Position::make(),
Text::make('Title'),
Text::make('Value'),
Switcher::make('Active')
])
];
}
//...
use MoonShine\Fields\Json;
use MoonShine\Fields\Position;
use MoonShine\Fields\Switcher;
use MoonShine\Fields\Text;
//...
public function fields(): array
{
return [
Json::make('Product Options', 'options')
->fields([
Position::make(),
Text::make('Title'),
Text::make('Value'),
Switcher::make('Active')
])
];
}
//...
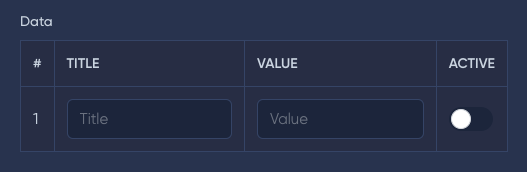
Sometimes you only need to store values in the database. To do this, you can use the onlyValue()
method. The result will be json ['value']
.
onlyValue(string $value = 'Value')
onlyValue(string $value = 'Value')
onlyValue(string $value = 'Value')
onlyValue(string $value = 'Value')
onlyValue(string $value = 'Value')
use MoonShine\Fields\Json;
public function fields(): array
{
return [
Json::make('Data')
->onlyValue()
];
}
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->onlyValue()
];
}
//...
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->onlyValue()
];
}
//...
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->onlyValue()
];
}
//...
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->onlyValue()
];
}
//...
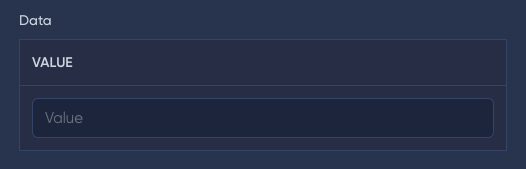
You can use the default()
method if you need to specify a default value for a field.
default(mixed $default)
default(mixed $default)
default(mixed $default)
default(mixed $default)
default(mixed $default)
use MoonShine\Fields\Json;
use MoonShine\Fields\Switcher;
use MoonShine\Fields\Text;
public function fields(): array
{
return [
Json::make('Data')
->keyValue('Key', 'Value')
->default([
[
'key' => 'Default key',
'value' => 'Default value'
]
]),
Json::make('Product Options', 'options')
->fields([
Text::make('Title'),
Text::make('Value'),
Switcher::make('Active')
])
->default([
[
'title' => 'Default title',
'value' => 'Default value',
'active' => true
]
]),
Json::make('Values')
->onlyValue()
->default([
['value' => 'Default value']
])
];
}
use MoonShine\Fields\Json;
use MoonShine\Fields\Switcher;
use MoonShine\Fields\Text;
//...
public function fields(): array
{
return [
Json::make('Data')
->keyValue('Key', 'Value')
->default([
[
'key' => 'Default key',
'value' => 'Default value'
]
]),
Json::make('Product Options', 'options')
->fields([
Text::make('Title'),
Text::make('Value'),
Switcher::make('Active')
])
->default([
[
'title' => 'Default title',
'value' => 'Default value',
'active' => true
]
]),
Json::make('Values')
->onlyValue()
->default([
['value' => 'Default value']
])
];
}
//...
use MoonShine\Fields\Json;
use MoonShine\Fields\Switcher;
use MoonShine\Fields\Text;
//...
public function fields(): array
{
return [
Json::make('Data')
->keyValue('Key', 'Value')
->default([
[
'key' => 'Default key',
'value' => 'Default value'
]
]),
Json::make('Product Options', 'options')
->fields([
Text::make('Title'),
Text::make('Value'),
Switcher::make('Active')
])
->default([
[
'title' => 'Default title',
'value' => 'Default value',
'active' => true
]
]),
Json::make('Values')
->onlyValue()
->default([
['value' => 'Default value']
])
];
}
//...
use MoonShine\Fields\Json;
use MoonShine\Fields\Switcher;
use MoonShine\Fields\Text;
//...
public function fields(): array
{
return [
Json::make('Data')
->keyValue('Key', 'Value')
->default([
[
'key' => 'Default key',
'value' => 'Default value'
]
]),
Json::make('Product Options', 'options')
->fields([
Text::make('Title'),
Text::make('Value'),
Switcher::make('Active')
])
->default([
[
'title' => 'Default title',
'value' => 'Default value',
'active' => true
]
]),
Json::make('Values')
->onlyValue()
->default([
['value' => 'Default value']
])
];
}
//...
use MoonShine\Fields\Json;
use MoonShine\Fields\Switcher;
use MoonShine\Fields\Text;
//...
public function fields(): array
{
return [
Json::make('Data')
->keyValue('Key', 'Value')
->default([
[
'key' => 'Default key',
'value' => 'Default value'
]
]),
Json::make('Product Options', 'options')
->fields([
Text::make('Title'),
Text::make('Value'),
Switcher::make('Active')
])
->default([
[
'title' => 'Default title',
'value' => 'Default value',
'active' => true
]
]),
Json::make('Values')
->onlyValue()
->default([
['value' => 'Default value']
])
];
}
//...
By default, the Json field contains only one entry. The creatable()
method allows you to add entries, and the removable()
method allows you to remove existing ones.
creatable(
Closure|bool|null $condition = null,
?int $limit = null,
?ActionButton $button = null
)
creatable(
Closure|bool|null $condition = null,
?int $limit = null,
?ActionButton $button = null
)
creatable(
Closure|bool|null $condition = null,
?int $limit = null,
?ActionButton $button = null
)
creatable(
Closure|bool|null $condition = null,
?int $limit = null,
?ActionButton $button = null
)
creatable(
Closure|bool|null $condition = null,
?int $limit = null,
?ActionButton $button = null
)
-$condition
- method execution condition,
-$limit
- number of records that can be added,
-$button
- custom add button.
removable(
Closure|bool|null $condition = null,
array $attributes = []
)
removable(
Closure|bool|null $condition = null,
array $attributes = []
)
removable(
Closure|bool|null $condition = null,
array $attributes = []
)
removable(
Closure|bool|null $condition = null,
array $attributes = []
)
removable(
Closure|bool|null $condition = null,
array $attributes = []
)
-$condition
- condition for executing the method,
-$attributes
- additional button attributes.
use MoonShine\Fields\Json;
public function fields(): array
{
return [
Json::make('Data')
->keyValue()
->creatable(limit: 6)
->removable()
];
}
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->keyValue()
->creatable(limit: 6)
->removable()
];
}
//...
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->keyValue()
->creatable(limit: 6)
->removable()
];
}
//...
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->keyValue()
->creatable(limit: 6)
->removable()
];
}
//...
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->keyValue()
->creatable(limit: 6)
->removable()
];
}
//...
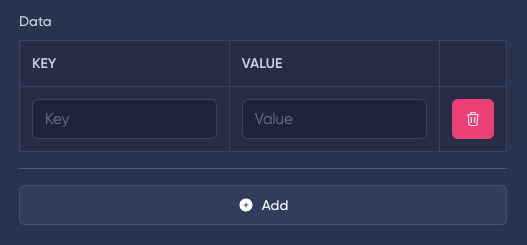
Custom add button
use MoonShine\Fields\Json;
public function fields(): array
{
return [
Json::make('Data')
->keyValue()
->creatable(
button: ActionButton::make('New', '#')->primary()
)
];
}
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->keyValue()
->creatable(
button: ActionButton::make('New', '#')->primary()
)
];
}
//...
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->keyValue()
->creatable(
button: ActionButton::make('New', '#')->primary()
)
];
}
//...
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->keyValue()
->creatable(
button: ActionButton::make('New', '#')->primary()
)
];
}
//...
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->keyValue()
->creatable(
button: ActionButton::make('New', '#')->primary()
)
];
}
//...
Attributes for the delete button
use MoonShine\Fields\Json;
public function fields(): array
{
return [
Json::make('Data', 'data.content')->fields([
Text::make('Title'),
Image::make('Image'),
Text::make('Value'),
])
->removable(attributes: ['@click.prevent' => 'customAsyncRemove'])
->creatable()
];
}
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data', 'data.content')->fields([
Text::make('Title'),
Image::make('Image'),
Text::make('Value'),
])
->removable(attributes: ['@click.prevent' => 'customAsyncRemove'])
->creatable()
];
}
//...
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data', 'data.content')->fields([
Text::make('Title'),
Image::make('Image'),
Text::make('Value'),
])
->removable(attributes: ['@click.prevent' => 'customAsyncRemove'])
->creatable()
];
}
//...
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data', 'data.content')->fields([
Text::make('Title'),
Image::make('Image'),
Text::make('Value'),
])
->removable(attributes: ['@click.prevent' => 'customAsyncRemove'])
->creatable()
];
}
//...
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data', 'data.content')->fields([
Text::make('Title'),
Image::make('Image'),
Text::make('Value'),
])
->removable(attributes: ['@click.prevent' => 'customAsyncRemove'])
->creatable()
];
}
//...
You can get nested values of JSON fields using .
.
Values can be edited, but the changes will not affect other keys.
{"info": [{"title": "Info title", "value": "Info value"}], "content": [{"title": "Content title", "value": "Content value"}]}
{"info": [{"title": "Info title", "value": "Info value"}], "content": [{"title": "Content title", "value": "Content value"}]}
{"info": [{"title": "Info title", "value": "Info value"}], "content": [{"title": "Content title", "value": "Content value"}]}
{"info": [{"title": "Info title", "value": "Info value"}], "content": [{"title": "Content title", "value": "Content value"}]}
{"info": [{"title": "Info title", "value": "Info value"}], "content": [{"title": "Content title", "value": "Content value"}]}
use MoonShine\Fields\Json;
public function fields(): array
{
return [
Json::make('Data', 'data.content')
->fields([
Text::make('Title'),
Text::make('Value'),
])->removable()
];
}
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data', 'data.content')
->fields([
Text::make('Title'),
Text::make('Value'),
])->removable()
];
}
//...
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data', 'data.content')
->fields([
Text::make('Title'),
Text::make('Value'),
])->removable()
];
}
//...
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data', 'data.content')
->fields([
Text::make('Title'),
Text::make('Value'),
])->removable()
];
}
//...
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data', 'data.content')
->fields([
Text::make('Title'),
Text::make('Value'),
])->removable()
];
}
//...
The vertical()
method allows you to change the horizontal layout of the fields to vertical.
vertical()
vertical()
vertical()
vertical()
vertical()
use MoonShine\Fields\Json;
public function fields(): array
{
return [
Json::make('Data')
->keyValue()
->vertical()
];
}
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->keyValue()
->vertical()
];
}
//...
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->keyValue()
->vertical()
];
}
//...
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->keyValue()
->vertical()
];
}
//...
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->keyValue()
->vertical()
];
}
//...
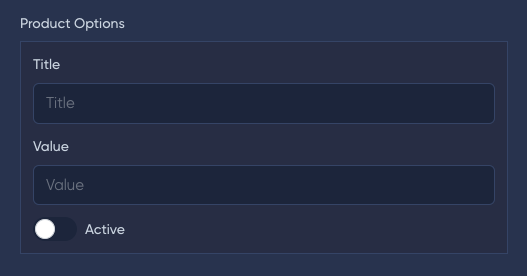
The Json field can work with relationships; the asRelation()
method is used for this, to which you need to assign ModelResource relationships and specify an array of editable fields.
asRelation(ModelResource $resource)
asRelation(ModelResource $resource)
asRelation(ModelResource $resource)
asRelation(ModelResource $resource)
asRelation(ModelResource $resource)
use MoonShine\Fields\ID;
use MoonShine\Fields\Json;
use MoonShine\Fields\Relationships\BelongsTo;
use MoonShine\Fields\Text;
public function fields(): array
{
return [
Json::make('Comments', 'comments')
->asRelation(new CommentResource())
->fields([
ID::make(),
BelongsTo::make('Article')
->setColumn('article_id')
->searchable(),
BelongsTo::make('User')
->setColumn('user_id'),
Text::make('Text')->required(),
])
->creatable()
->removable()
];
}
use MoonShine\Fields\ID;
use MoonShine\Fields\Json;
use MoonShine\Fields\Relationships\BelongsTo;
use MoonShine\Fields\Text;
//...
public function fields(): array
{
return [
Json::make('Comments', 'comments')
->asRelation(new CommentResource())
->fields([
ID::make(),
BelongsTo::make('Article')
->setColumn('article_id')
->searchable(),
BelongsTo::make('User')
->setColumn('user_id'),
Text::make('Text')->required(),
])
->creatable()
->removable()
];
}
//...
use MoonShine\Fields\ID;
use MoonShine\Fields\Json;
use MoonShine\Fields\Relationships\BelongsTo;
use MoonShine\Fields\Text;
//...
public function fields(): array
{
return [
Json::make('Comments', 'comments')
->asRelation(new CommentResource())
->fields([
ID::make(),
BelongsTo::make('Article')
->setColumn('article_id')
->searchable(),
BelongsTo::make('User')
->setColumn('user_id'),
Text::make('Text')->required(),
])
->creatable()
->removable()
];
}
//...
use MoonShine\Fields\ID;
use MoonShine\Fields\Json;
use MoonShine\Fields\Relationships\BelongsTo;
use MoonShine\Fields\Text;
//...
public function fields(): array
{
return [
Json::make('Comments', 'comments')
->asRelation(new CommentResource())
->fields([
ID::make(),
BelongsTo::make('Article')
->setColumn('article_id')
->searchable(),
BelongsTo::make('User')
->setColumn('user_id'),
Text::make('Text')->required(),
])
->creatable()
->removable()
];
}
//...
use MoonShine\Fields\ID;
use MoonShine\Fields\Json;
use MoonShine\Fields\Relationships\BelongsTo;
use MoonShine\Fields\Text;
//...
public function fields(): array
{
return [
Json::make('Comments', 'comments')
->asRelation(new CommentResource())
->fields([
ID::make(),
BelongsTo::make('Article')
->setColumn('article_id')
->searchable(),
BelongsTo::make('User')
->setColumn('user_id'),
Text::make('Text')->required(),
])
->creatable()
->removable()
];
}
//...
For relationships, the presence of the ID field in the fields method is mandatory!
When using BelongsTo it is necessary to use the method setColumn()
set a field in a database table!
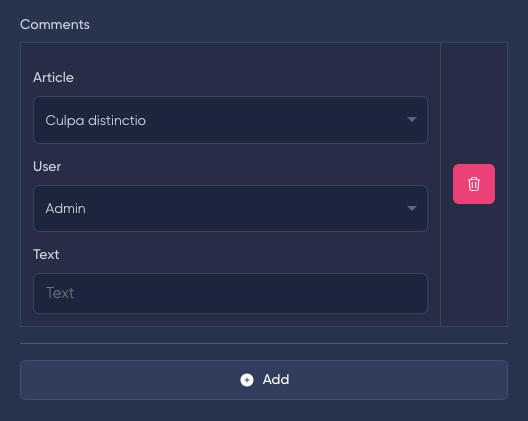
If the field is used to build a filter, then you must use the filterMode()
method. This method adapts the behavior of the field and sets creatable = false
.
use MoonShine\Fields\Json;
use MoonShine\Fields\Text;
public function filters(): array
{
return [
Json::make('Data')
->fields([
Text::make('Title', 'title'),
Text::make('Value', 'value')
])
->filterMode()
];
}
use MoonShine\Fields\Json;
use MoonShine\Fields\Text;
//...
public function filters(): array
{
return [
Json::make('Data')
->fields([
Text::make('Title', 'title'),
Text::make('Value', 'value')
])
->filterMode()
];
}
//...
use MoonShine\Fields\Json;
use MoonShine\Fields\Text;
//...
public function filters(): array
{
return [
Json::make('Data')
->fields([
Text::make('Title', 'title'),
Text::make('Value', 'value')
])
->filterMode()
];
}
//...
use MoonShine\Fields\Json;
use MoonShine\Fields\Text;
//...
public function filters(): array
{
return [
Json::make('Data')
->fields([
Text::make('Title', 'title'),
Text::make('Value', 'value')
])
->filterMode()
];
}
//...
use MoonShine\Fields\Json;
use MoonShine\Fields\Text;
//...
public function filters(): array
{
return [
Json::make('Data')
->fields([
Text::make('Title', 'title'),
Text::make('Value', 'value')
])
->filterMode()
];
}
//...
The buttons()
method allows you to add additional buttons to the Json field.
buttons(array $buttons)
buttons(array $buttons)
buttons(array $buttons)
buttons(array $buttons)
buttons(array $buttons)
use MoonShine\ActionButtons\ActionButton;
use MoonShine\Fields\Json;
public function fields(): array
{
return [
Json::make('Data', 'data.content')->fields([
Text::make('Title'),
Image::make('Image'),
Text::make('Value'),
])->buttons([
ActionButton::make('', '#')
->icon('heroicons.outline.trash')
->onClick(fn() => 'remove()', 'prevent')
->customAttributes(['class' => 'btn-secondary'])
->showInLine()
])
];
}
use MoonShine\ActionButtons\ActionButton;
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data', 'data.content')->fields([
Text::make('Title'),
Image::make('Image'),
Text::make('Value'),
])->buttons([
ActionButton::make('', '#')
->icon('heroicons.outline.trash')
->onClick(fn() => 'remove()', 'prevent')
->customAttributes(['class' => 'btn-secondary'])
->showInLine()
])
];
}
//...
use MoonShine\ActionButtons\ActionButton;
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data', 'data.content')->fields([
Text::make('Title'),
Image::make('Image'),
Text::make('Value'),
])->buttons([
ActionButton::make('', '#')
->icon('heroicons.outline.trash')
->onClick(fn() => 'remove()', 'prevent')
->customAttributes(['class' => 'btn-secondary'])
->showInLine()
])
];
}
//...
use MoonShine\ActionButtons\ActionButton;
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data', 'data.content')->fields([
Text::make('Title'),
Image::make('Image'),
Text::make('Value'),
])->buttons([
ActionButton::make('', '#')
->icon('heroicons.outline.trash')
->onClick(fn() => 'remove()', 'prevent')
->customAttributes(['class' => 'btn-secondary'])
->showInLine()
])
];
}
//...
use MoonShine\ActionButtons\ActionButton;
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data', 'data.content')->fields([
Text::make('Title'),
Image::make('Image'),
Text::make('Value'),
])->buttons([
ActionButton::make('', '#')
->icon('heroicons.outline.trash')
->onClick(fn() => 'remove()', 'prevent')
->customAttributes(['class' => 'btn-secondary'])
->showInLine()
])
];
}
//...
The Json field has methods with which you can modify the delete button or change TableBuilder for preview and form.
modifyRemoveButton()
The modifyRemoveButton()
method allows you to change the remove button.
modifyRemoveButton(Closure $callback)
/**
* @param Closure(ActionButton $button, self $field): ActionButton $callback
*/
modifyRemoveButton(Closure $callback)
/**
* @param Closure(ActionButton $button, self $field): ActionButton $callback
*/
modifyRemoveButton(Closure $callback)
/**
* @param Closure(ActionButton $button, self $field): ActionButton $callback
*/
modifyRemoveButton(Closure $callback)
/**
* @param Closure(ActionButton $button, self $field): ActionButton $callback
*/
modifyRemoveButton(Closure $callback)
use MoonShine\ActionButtons\ActionButton;
use MoonShine\Fields\Json;
public function fields(): array
{
return [
Json::make('Data')
->modifyRemoveButton(
fn(ActionButton $button) => $button->customAttributes([
'class' => 'btn-secondary'
])
)
];
}
use MoonShine\ActionButtons\ActionButton;
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->modifyRemoveButton(
fn(ActionButton $button) => $button->customAttributes([
'class' => 'btn-secondary'
])
)
];
}
//...
use MoonShine\ActionButtons\ActionButton;
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->modifyRemoveButton(
fn(ActionButton $button) => $button->customAttributes([
'class' => 'btn-secondary'
])
)
];
}
//...
use MoonShine\ActionButtons\ActionButton;
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->modifyRemoveButton(
fn(ActionButton $button) => $button->customAttributes([
'class' => 'btn-secondary'
])
)
];
}
//...
use MoonShine\ActionButtons\ActionButton;
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->modifyRemoveButton(
fn(ActionButton $button) => $button->customAttributes([
'class' => 'btn-secondary'
])
)
];
}
//...
modifyTable()
The modifyTable()
method allows you to change the TableBuilder for the preview and form.
modifyTable(Closure $callback)
/**
* @param Closure(TableBuilder $table, bool $preview): TableBuilder $callback
*/
modifyTable(Closure $callback)
/**
* @param Closure(TableBuilder $table, bool $preview): TableBuilder $callback
*/
modifyTable(Closure $callback)
/**
* @param Closure(TableBuilder $table, bool $preview): TableBuilder $callback
*/
modifyTable(Closure $callback)
/**
* @param Closure(TableBuilder $table, bool $preview): TableBuilder $callback
*/
modifyTable(Closure $callback)
use MoonShine\Components\TableBuilder;
use MoonShine\Fields\Json;
public function fields(): array
{
return [
Json::make('Data')
->modifyTable(
fn(TableBuilder $table, bool $preview) => $table->customAttributes([
'style' => 'width: 50%;'
])
)
];
}
use MoonShine\Components\TableBuilder;
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->modifyTable(
fn(TableBuilder $table, bool $preview) => $table->customAttributes([
'style' => 'width: 50%;'
])
)
];
}
//...
use MoonShine\Components\TableBuilder;
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->modifyTable(
fn(TableBuilder $table, bool $preview) => $table->customAttributes([
'style' => 'width: 50%;'
])
)
];
}
//...
use MoonShine\Components\TableBuilder;
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->modifyTable(
fn(TableBuilder $table, bool $preview) => $table->customAttributes([
'style' => 'width: 50%;'
])
)
];
}
//...
use MoonShine\Components\TableBuilder;
use MoonShine\Fields\Json;
//...
public function fields(): array
{
return [
Json::make('Data')
->modifyTable(
fn(TableBuilder $table, bool $preview) => $table->customAttributes([
'style' => 'width: 50%;'
])
)
];
}
//...