# Basics
The Json field includes all the base methods.
Json has several methods to set the field structure:
keyValue()
, onlyValue()
and fields()
.
In the database, the field must be of text or json type. Also cast eloquent of an array or json or collection model.
# Key/Value
The easiest way to work with a Json field is to use the keyValue()
method.
The result will be a simple json {key: value}
.
keyValue( string $key = 'Key', string $value = 'Value')
use MoonShine\Fields\Json; //... public function fields(): array{ return [ Json::make('Data') ->keyValue() ];} //...

The default keys and values are the Text field, but you can use other fields for primitive data.
use MoonShine\Fields\Json; use MoonShine\Fields\Select; //... public function fields(): array{ return [ Json::make('Label', 'data')->keyValue( keyField: Select::make('Key')->options(['vk' => 'VK', 'email' => 'E-mail']), valueField: Select::make('Value')->options(['1' => '1', '2' => '2']), ), ];} //...
# With a set of fields
For more advanced use, use the fields()
method
and pass the required set of fields.
As a result, the following json will be generated
[{title: 'title', value: 'value', active: 'active'}]
fields(Fields|Closure|array $fields)
use MoonShine\Fields\Json; use MoonShine\Fields\Position; use MoonShine\Fields\Switcher; use MoonShine\Fields\Text; //... public function fields(): array{ return [ Json::make('Product Options', 'options') ->fields([ Position::make(), Text::make('Title'), Text::make('Value'), Switcher::make('Active') ]) ];} //...
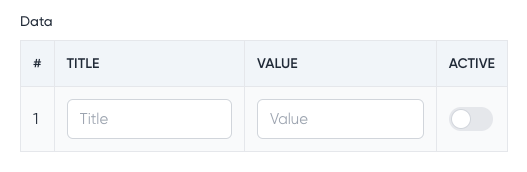
# Meaning only
Sometimes you only need to store values in the database,
To do this, you can use the onlyValue()
method.
The result will be json ['value']
.
onlyValue(string $value = 'Value')
use MoonShine\Fields\Json; //... public function fields(): array{ return [ Json::make('Data') ->onlyValue() ];} //...
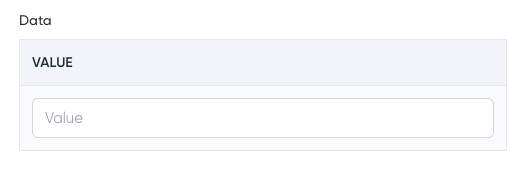
# Default value
You can use the default()
method if you need to specify a default value for a field.
default(mixed $default)
use MoonShine\Fields\Json;use MoonShine\Fields\Switcher;use MoonShine\Fields\Text; //... public function fields(): array{ return [ Json::make('Data') ->keyValue('Key', 'Value') ->default([ [ 'key' => 'Default key', 'value' => 'Default value' ] ]), Json::make('Product Options', 'options') ->fields([ Text::make('Title'), Text::make('Value'), Switcher::make('Active') ]) ->default([ [ 'title' => 'Default title', 'value' => 'Default value', 'active' => true ] ]), Json::make('Values') ->onlyValue() ->default([ ['value' => 'Default value'] ]) ];} //...
# Add/Remove
By default, the Json field contains only one entry,
The creatable()
method allows you to add entries,
and the removable()
method allows you to remove existing ones.
creatable( Closure|bool|null $condition = null, ?int $limit = null, ?ActionButton $button = null)
$condition
- method execution condition,$limit
- number of records that can be added,$button
- custom add button.
removable( Closure|bool|null $condition = null, array $attributes = [])
$condition
- condition for executing the method,$attributes
- additional button attributes.
use MoonShine\Fields\Json; //... public function fields(): array{ return [ Json::make('Data') ->keyValue() ->creatable(limit: 6) ->removable() ];} //...

use MoonShine\Fields\Json; //... public function fields(): array{ return [ Json::make('Data') ->keyValue() ->creatable( button: ActionButton::make('New', '#')->primary() ) ];} //...
use MoonShine\Fields\Json; //... public function fields(): array{ return [ Json::make('Data', 'data.content')->fields([ Text::make('Title'), Image::make('Image'), Text::make('Value'), ]) ->removable(attributes: ['@click.prevent' => 'customAsyncRemove']) ->creatable() ];} //...
# Nested values
You can get nested values of JSON fields using .
.
Values can be edited, but the changes will not affect other keys.
{"info": [{"title": "Info title", "value": "Info value"}], "content": [{"title": "Content title", "value": "Content value"}]}
use MoonShine\Fields\Json; //... public function fields(): array{ return [ Json::make('Data', 'data.content') ->fields([ Text::make('Title'), Text::make('Value'), ])->removable() ];} //...
# Vertical display
The vertical()
method allows you to change the horizontal layout of the fields to vertical.
vertical()
use MoonShine\Fields\Json; //... public function fields(): array{ return [ Json::make('Data') ->keyValue() ->vertical() ];} //...
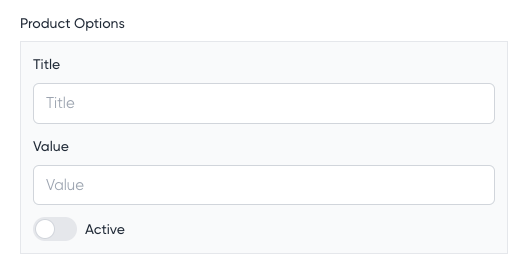
# Relationships via Json
The Json field can work with relationships; the asRelation()
method is used for this,
to which you need to assign ModelResource relationships and specify an array of editable fields.
asRelation(ModelResource $resource)
use MoonShine\Fields\ID;use MoonShine\Fields\Json;use MoonShine\Fields\Relationships\BelongsTo;use MoonShine\Fields\Text; //... public function fields(): array{ return [ Json::make('Comments', 'comments') ->asRelation(new CommentResource()) ->fields([ ID::make(), BelongsTo::make('Article') ->setColumn('article_id') ->searchable(), BelongsTo::make('User') ->setColumn('user_id'), Text::make('Text')->required(), ]) ->creatable() ->removable() ];} //...
For relationships, the presence of the ID field in the fields method is mandatory!
When using BelongsTo it is necessary to use the method
setColumn()
set a field in a database table!
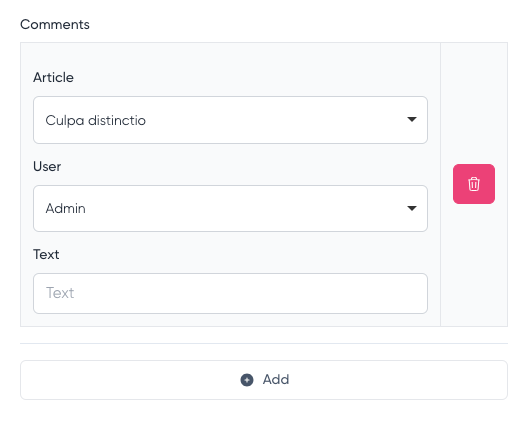
# Filter
If the field is used to build a filter, then you must use the filterMode()
method.
This method adapts the behavior of the field and sets creatable = false
.
use MoonShine\Fields\Json;use MoonShine\Fields\Text; //... public function filters(): array{ return [ Json::make('Data') ->fields([ Text::make('Title', 'title'), Text::make('Value', 'value') ]) ->filterMode() ];} //...
# Buttons
The buttons()
method allows you to add additional buttons to the Json field.
buttons(array $buttons)
use MoonShine\ActionButtons\ActionButton;use MoonShine\Fields\Json; //... public function fields(): array{ return [ Json::make('Data', 'data.content')->fields([ Text::make('Title'), Image::make('Image'), Text::make('Value'), ])->buttons([ ActionButton::make('', '#') ->icon('heroicons.outline.trash') ->onClick(fn() => 'remove()', 'prevent') ->customAttributes(['class' => 'btn-secondary']) ->showInLine() ]) ];} //...