Extends
Number
* has the same features
# Make
The Range field is an extension of Number, allows you to set values for two logically related fields.
Since the range has two values, you need to specify them using the fromTo()
method.
fromTo(string $fromField, string $toField)
use MoonShine\Fields\Range; //... public function fields(): array{ return [ Range::make('Age') ->fromTo('age_from', 'age_to') ];} //...
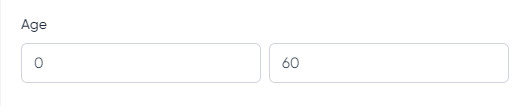
# Attributes
If you need to add custom attributes for fields, you can use the appropriate methods
fromAttributes()
and toAttributes()
.
fromAttributes(array $attributes)
toAttributes(array $attributes)
use MoonShine\Fields\Range; //... public function fields(): array{ return [ Range::make('Age') ->fromTo('age_from', 'age_to') ->fromAttributes(['placeholder'=> 'from']) ->toAttributes(['placeholder'=> 'to']) ];} //...
# Filter
While using the Range field to construct a filter, method fromTo()
is not used,
because filtering occurs on one field in the database table.
use MoonShine\Fields\Range; //... public function filters(): array{ return [ Range::make('Age', 'age') ];} //...