Validation is as easy as in the FormRequests
classes from Laravel.
It is enough to add rules in the model resource rules()
method in the usual manner.
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Fields\Text;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
public function rules($item): array
{
return [
'title' => ['required', 'string', 'min:5']
];
}
}
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Fields\Text;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
//...
public function rules($item): array
{
return [
'title' => ['required', 'string', 'min:5']
];
}
//...
}
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Fields\Text;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
//...
public function rules($item): array
{
return [
'title' => ['required', 'string', 'min:5']
];
}
//...
}
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Fields\Text;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
//...
public function rules($item): array
{
return [
'title' => ['required', 'string', 'min:5']
];
}
//...
}
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Fields\Text;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
//...
public function rules($item): array
{
return [
'title' => ['required', 'string', 'min:5']
];
}
//...
}
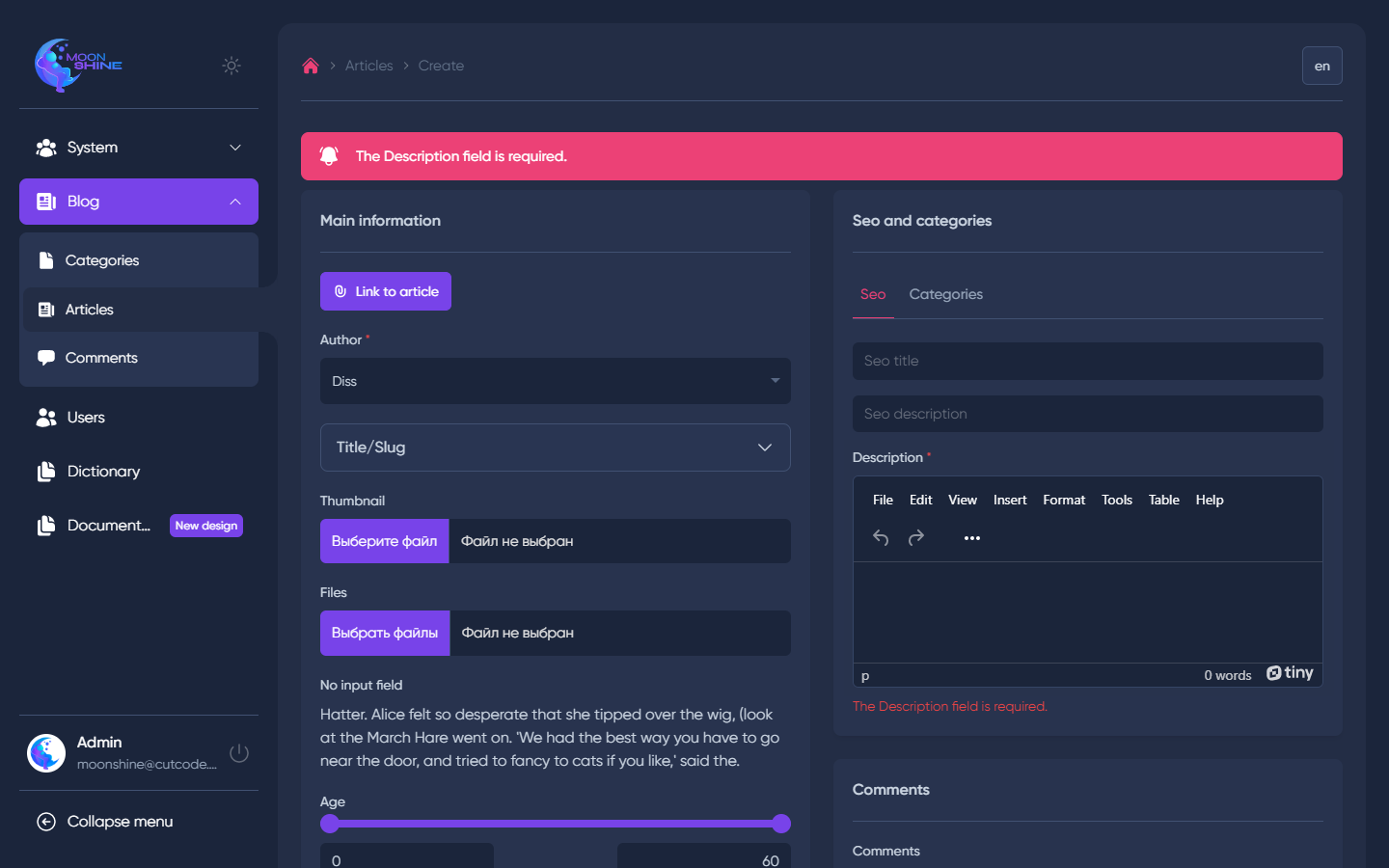
Using the validationMessages()
method you can create your own validation error messages.
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Fields\Text;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
public function validationMessages(): array
{
return [
'email.required' => 'Required email'
];
}
}
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Fields\Text;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
//...
public function validationMessages(): array
{
return [
'email.required' => 'Required email'
];
}
//...
}
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Fields\Text;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
//...
public function validationMessages(): array
{
return [
'email.required' => 'Required email'
];
}
//...
}
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Fields\Text;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
//...
public function validationMessages(): array
{
return [
'email.required' => 'Required email'
];
}
//...
}
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Fields\Text;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
//...
public function validationMessages(): array
{
return [
'email.required' => 'Required email'
];
}
//...
}
If you need to prepare or clean up any data from the request before applying your validation rules, you can use the prepareForValidation()
method.
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Fields\Text;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
public function prepareForValidation(): void
{
moonshineRequest()?->merge([
'email' => request()
?->string('email')
->lower()
->value()
]);
}
}
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Fields\Text;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
//...
public function prepareForValidation(): void
{
moonshineRequest()?->merge([
'email' => request()
?->string('email')
->lower()
->value()
]);
}
//...
}
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Fields\Text;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
//...
public function prepareForValidation(): void
{
moonshineRequest()?->merge([
'email' => request()
?->string('email')
->lower()
->value()
]);
}
//...
}
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Fields\Text;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
//...
public function prepareForValidation(): void
{
moonshineRequest()?->merge([
'email' => request()
?->string('email')
->lower()
->value()
]);
}
//...
}
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Fields\Text;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
//...
public function prepareForValidation(): void
{
moonshineRequest()?->merge([
'email' => request()
?->string('email')
->lower()
->value()
]);
}
//...
}
To add buttons, use ActionButton and the FormButtons
or buttons
methods in the resource.
More details ActionButton
public function formButtons(): array
{
return [
ActionButton::make('Link', '/endpoint'),
];
}
public function formButtons(): array
{
return [
ActionButton::make('Link', '/endpoint'),
];
}
public function formButtons(): array
{
return [
ActionButton::make('Link', '/endpoint'),
];
}
public function formButtons(): array
{
return [
ActionButton::make('Link', '/endpoint'),
];
}
public function formButtons(): array
{
return [
ActionButton::make('Link', '/endpoint'),
];
}
You can also use the buttons
method, but in this case, the buttons will be on the resource all other pages.
public function buttons(): array
{
return [
ActionButton::make('Link', '/endpoint'),
];
}
public function buttons(): array
{
return [
ActionButton::make('Link', '/endpoint'),
];
}
public function buttons(): array
{
return [
ActionButton::make('Link', '/endpoint'),
];
}
public function buttons(): array
{
return [
ActionButton::make('Link', '/endpoint'),
];
}
public function buttons(): array
{
return [
ActionButton::make('Link', '/endpoint'),
];
}
Switch mode without reboot to save data.
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
protected bool $isAsync = true;
}
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
protected bool $isAsync = true;
// ...
}
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
protected bool $isAsync = true;
// ...
}
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
protected bool $isAsync = true;
// ...
}
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
protected bool $isAsync = true;
// ...
}
Switch form mode to Precognitive validation.
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
protected bool $isPrecognitive = true;
}
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
protected bool $isPrecognitive = true;
// ...
}
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
protected bool $isPrecognitive = true;
// ...
}
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
protected bool $isPrecognitive = true;
// ...
}
namespace App\MoonShine\Resources;
use App\Models\Post;
use MoonShine\Resources\ModelResource;
class PostResource extends ModelResource
{
protected string $model = Post::class;
protected string $title = 'Posts';
protected bool $isPrecognitive = true;
// ...
}