The Sidebar system component is used to create a side menu in MoonShine.
You can create a Sidebar using the static method make()
class Sidebar
.
make(array $components = [])
make(array $components = [])
make(array $components = [])
make(array $components = [])
make(array $components = [])
The make()
method takes an array of components as a parameter.
namespace App\MoonShine;
use MoonShine\Components\Layout\LayoutBlock;
use MoonShine\Components\Layout\LayoutBuilder;
use MoonShine\Components\Layout\Menu;
use MoonShine\Components\Layout\Profile;
use MoonShine\Components\Layout\Sidebar;
use MoonShine\Contracts\MoonShineLayoutContract;
final class MoonShineLayout implements MoonShineLayoutContract
{
public static function build(): LayoutBuilder
{
return LayoutBuilder::make([
Sidebar::make([
Menu::make()->customAttributes(['class' => 'mt-2']),
Profile::make(withBorder: true)
]),
]);
}
}
namespace App\MoonShine;
use MoonShine\Components\Layout\LayoutBlock;
use MoonShine\Components\Layout\LayoutBuilder;
use MoonShine\Components\Layout\Menu;
use MoonShine\Components\Layout\Profile;
use MoonShine\Components\Layout\Sidebar;
use MoonShine\Contracts\MoonShineLayoutContract;
final class MoonShineLayout implements MoonShineLayoutContract
{
public static function build(): LayoutBuilder
{
return LayoutBuilder::make([
Sidebar::make([
Menu::make()->customAttributes(['class' => 'mt-2']),
Profile::make(withBorder: true)
]),
//...
]);
}
}
namespace App\MoonShine;
use MoonShine\Components\Layout\LayoutBlock;
use MoonShine\Components\Layout\LayoutBuilder;
use MoonShine\Components\Layout\Menu;
use MoonShine\Components\Layout\Profile;
use MoonShine\Components\Layout\Sidebar;
use MoonShine\Contracts\MoonShineLayoutContract;
final class MoonShineLayout implements MoonShineLayoutContract
{
public static function build(): LayoutBuilder
{
return LayoutBuilder::make([
Sidebar::make([
Menu::make()->customAttributes(['class' => 'mt-2']),
Profile::make(withBorder: true)
]),
//...
]);
}
}
namespace App\MoonShine;
use MoonShine\Components\Layout\LayoutBlock;
use MoonShine\Components\Layout\LayoutBuilder;
use MoonShine\Components\Layout\Menu;
use MoonShine\Components\Layout\Profile;
use MoonShine\Components\Layout\Sidebar;
use MoonShine\Contracts\MoonShineLayoutContract;
final class MoonShineLayout implements MoonShineLayoutContract
{
public static function build(): LayoutBuilder
{
return LayoutBuilder::make([
Sidebar::make([
Menu::make()->customAttributes(['class' => 'mt-2']),
Profile::make(withBorder: true)
]),
//...
]);
}
}
namespace App\MoonShine;
use MoonShine\Components\Layout\LayoutBlock;
use MoonShine\Components\Layout\LayoutBuilder;
use MoonShine\Components\Layout\Menu;
use MoonShine\Components\Layout\Profile;
use MoonShine\Components\Layout\Sidebar;
use MoonShine\Contracts\MoonShineLayoutContract;
final class MoonShineLayout implements MoonShineLayoutContract
{
public static function build(): LayoutBuilder
{
return LayoutBuilder::make([
Sidebar::make([
Menu::make()->customAttributes(['class' => 'mt-2']),
Profile::make(withBorder: true)
]),
//...
]);
}
}
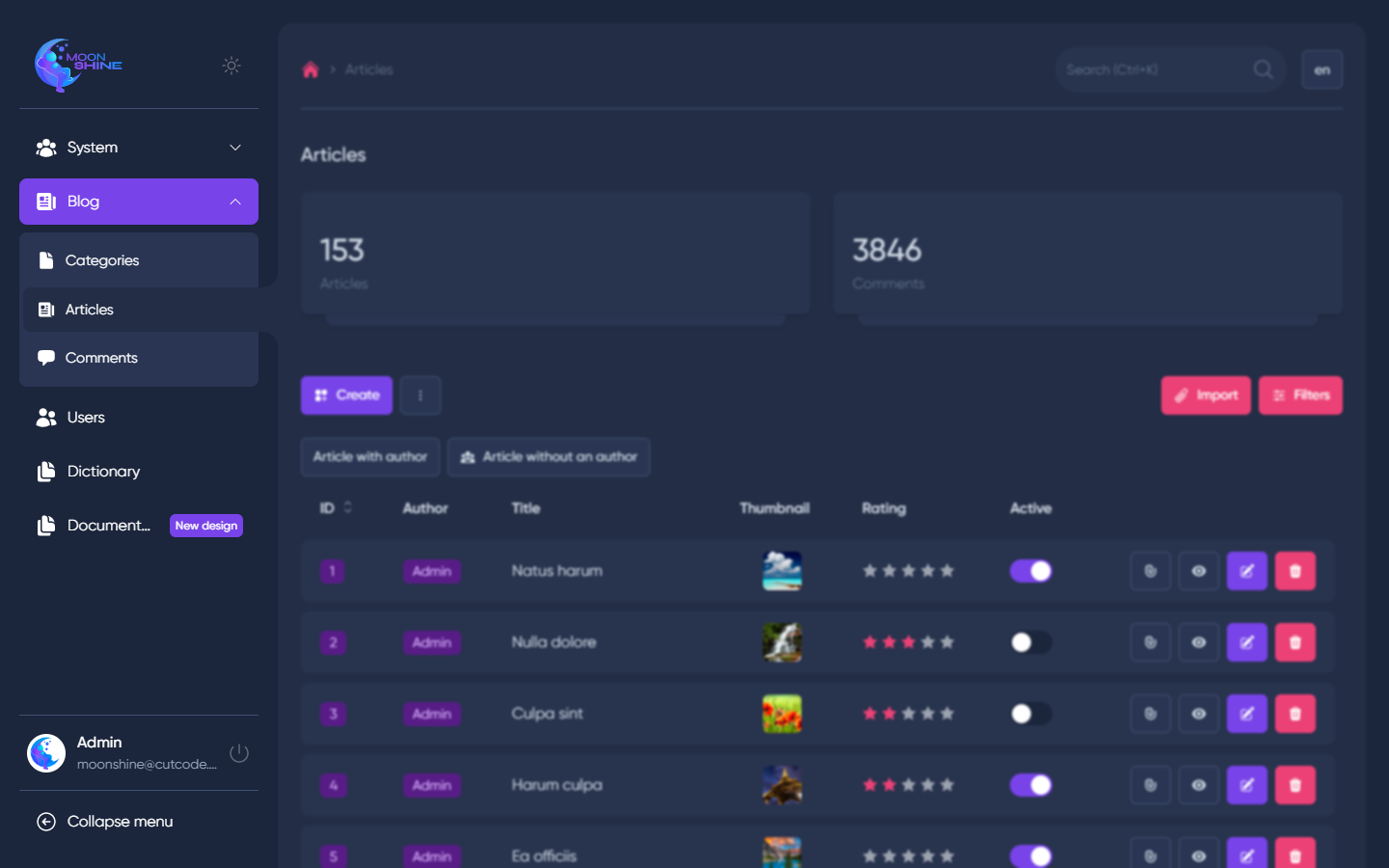
The hideLogo()
method allows you to hide the logo.
hideLogo()
hideLogo()
hideLogo()
hideLogo()
hideLogo()
namespace App\MoonShine;
use MoonShine\Components\Layout\LayoutBlock;
use MoonShine\Components\Layout\LayoutBuilder;
use MoonShine\Components\Layout\Menu;
use MoonShine\Components\Layout\Profile;
use MoonShine\Components\Layout\Sidebar;
use MoonShine\Contracts\MoonShineLayoutContract;
final class MoonShineLayout implements MoonShineLayoutContract
{
public static function build(): LayoutBuilder
{
return LayoutBuilder::make([
Sidebar::make([
Menu::make(),
Profile::make(withBorder: true)
])
->hideLogo(),
]);
}
}
namespace App\MoonShine;
use MoonShine\Components\Layout\LayoutBlock;
use MoonShine\Components\Layout\LayoutBuilder;
use MoonShine\Components\Layout\Menu;
use MoonShine\Components\Layout\Profile;
use MoonShine\Components\Layout\Sidebar;
use MoonShine\Contracts\MoonShineLayoutContract;
final class MoonShineLayout implements MoonShineLayoutContract
{
public static function build(): LayoutBuilder
{
return LayoutBuilder::make([
Sidebar::make([
Menu::make(),
Profile::make(withBorder: true)
])
->hideLogo(),
//...
]);
}
}
namespace App\MoonShine;
use MoonShine\Components\Layout\LayoutBlock;
use MoonShine\Components\Layout\LayoutBuilder;
use MoonShine\Components\Layout\Menu;
use MoonShine\Components\Layout\Profile;
use MoonShine\Components\Layout\Sidebar;
use MoonShine\Contracts\MoonShineLayoutContract;
final class MoonShineLayout implements MoonShineLayoutContract
{
public static function build(): LayoutBuilder
{
return LayoutBuilder::make([
Sidebar::make([
Menu::make(),
Profile::make(withBorder: true)
])
->hideLogo(),
//...
]);
}
}
namespace App\MoonShine;
use MoonShine\Components\Layout\LayoutBlock;
use MoonShine\Components\Layout\LayoutBuilder;
use MoonShine\Components\Layout\Menu;
use MoonShine\Components\Layout\Profile;
use MoonShine\Components\Layout\Sidebar;
use MoonShine\Contracts\MoonShineLayoutContract;
final class MoonShineLayout implements MoonShineLayoutContract
{
public static function build(): LayoutBuilder
{
return LayoutBuilder::make([
Sidebar::make([
Menu::make(),
Profile::make(withBorder: true)
])
->hideLogo(),
//...
]);
}
}
namespace App\MoonShine;
use MoonShine\Components\Layout\LayoutBlock;
use MoonShine\Components\Layout\LayoutBuilder;
use MoonShine\Components\Layout\Menu;
use MoonShine\Components\Layout\Profile;
use MoonShine\Components\Layout\Sidebar;
use MoonShine\Contracts\MoonShineLayoutContract;
final class MoonShineLayout implements MoonShineLayoutContract
{
public static function build(): LayoutBuilder
{
return LayoutBuilder::make([
Sidebar::make([
Menu::make(),
Profile::make(withBorder: true)
])
->hideLogo(),
//...
]);
}
}
The hideSwitcher()
method allows you to hide the theme switcher.
hideSwitcher()
hideSwitcher()
hideSwitcher()
hideSwitcher()
hideSwitcher()
namespace App\MoonShine;
use MoonShine\Components\Layout\LayoutBlock;
use MoonShine\Components\Layout\LayoutBuilder;
use MoonShine\Components\Layout\Menu;
use MoonShine\Components\Layout\Profile;
use MoonShine\Components\Layout\Sidebar;
use MoonShine\Contracts\MoonShineLayoutContract;
final class MoonShineLayout implements MoonShineLayoutContract
{
public static function build(): LayoutBuilder
{
return LayoutBuilder::make([
Sidebar::make([
Menu::make(),
Profile::make(withBorder: true)
])
->hideSwitcher(),
]);
}
}
namespace App\MoonShine;
use MoonShine\Components\Layout\LayoutBlock;
use MoonShine\Components\Layout\LayoutBuilder;
use MoonShine\Components\Layout\Menu;
use MoonShine\Components\Layout\Profile;
use MoonShine\Components\Layout\Sidebar;
use MoonShine\Contracts\MoonShineLayoutContract;
final class MoonShineLayout implements MoonShineLayoutContract
{
public static function build(): LayoutBuilder
{
return LayoutBuilder::make([
Sidebar::make([
Menu::make(),
Profile::make(withBorder: true)
])
->hideSwitcher(),
//...
]);
}
}
namespace App\MoonShine;
use MoonShine\Components\Layout\LayoutBlock;
use MoonShine\Components\Layout\LayoutBuilder;
use MoonShine\Components\Layout\Menu;
use MoonShine\Components\Layout\Profile;
use MoonShine\Components\Layout\Sidebar;
use MoonShine\Contracts\MoonShineLayoutContract;
final class MoonShineLayout implements MoonShineLayoutContract
{
public static function build(): LayoutBuilder
{
return LayoutBuilder::make([
Sidebar::make([
Menu::make(),
Profile::make(withBorder: true)
])
->hideSwitcher(),
//...
]);
}
}
namespace App\MoonShine;
use MoonShine\Components\Layout\LayoutBlock;
use MoonShine\Components\Layout\LayoutBuilder;
use MoonShine\Components\Layout\Menu;
use MoonShine\Components\Layout\Profile;
use MoonShine\Components\Layout\Sidebar;
use MoonShine\Contracts\MoonShineLayoutContract;
final class MoonShineLayout implements MoonShineLayoutContract
{
public static function build(): LayoutBuilder
{
return LayoutBuilder::make([
Sidebar::make([
Menu::make(),
Profile::make(withBorder: true)
])
->hideSwitcher(),
//...
]);
}
}
namespace App\MoonShine;
use MoonShine\Components\Layout\LayoutBlock;
use MoonShine\Components\Layout\LayoutBuilder;
use MoonShine\Components\Layout\Menu;
use MoonShine\Components\Layout\Profile;
use MoonShine\Components\Layout\Sidebar;
use MoonShine\Contracts\MoonShineLayoutContract;
final class MoonShineLayout implements MoonShineLayoutContract
{
public static function build(): LayoutBuilder
{
return LayoutBuilder::make([
Sidebar::make([
Menu::make(),
Profile::make(withBorder: true)
])
->hideSwitcher(),
//...
]);
}
}