Field depends on Eloquent Model
# Basics
Using this field you can generate a slug based on the selected field, and also store only unique values.
use MoonShine\Fields\Slug; //... public function fields(): array{ return [ Slug::make('Slug') ];} //...
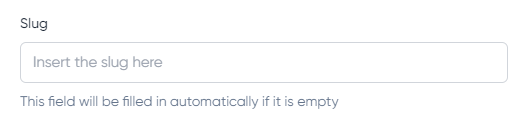
# Slug generation
Using the from()
method, you can specify based on which model field to generate a slug,
if there is no value.
from(string $from)
use MoonShine\Fields\Slug; //... public function fields(): array{ return [ Slug::make('Slug') ->from('title') ];} //...
# Delimiter
By default, -
is used as a word separator when generating a slug.
The separator()
method allows you to change this value.
separator(string $separator)
use MoonShine\Fields\Slug; //... public function fields(): array{ return [ Slug::make('Slug') ->separator('_') ];} //...
# Locale
By default, slug generation takes into account the specified application locale,
The locale()
method allows you to change this behavior for a field.
locale(string $local)
use MoonShine\Fields\Slug; //... public function fields(): array{ return [ Slug::make('Slug') ->locale('ru') ];} //...
# Unique value
If you need to save only unique slugs, you need to use the unique()
method.
unique()
use MoonShine\Fields\Slug; //... public function fields(): array{ return [ Slug::make('Slug') ->unique() ];} //...
# Live slug
The live()
method allows you to create a live field that will track changes to the original field.
use MoonShine\Fields\Slug;use MoonShine\Fields\Text; //... public function fields(): array{ return [ Text::make('Title') ->reactive(), Slug::make('Slug') ->from('title') ->live() ];} //...
Lives is based on field reactivity .