Buttons are displayed on resource pages: index page, form pages (create / edit), and detail page.
They are responsible for basic actions with elements and are components of ActionButton.
In MoonShine, there are many methods that allow you to override either a single button for the resource or an entire group.
The buttons for creating, viewing, editing, deleting, and mass deleting are placed in separate classes
to apply all necessary methods to them and thereby eliminate duplication, as these buttons are also used in HasMany
, BelongsToMany
, etc.
The modifyCreateButton()
method allows you to modify the button for creating a new item.
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyCreateButton(ActionButtonContract $button): ActionButtonContract
{
return $button->error();
}
namespaces
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyCreateButton(ActionButtonContract $button): ActionButtonContract
{
return $button->error();
}
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyCreateButton(ActionButtonContract $button): ActionButtonContract
{
return $button->error();
}
namespaces
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyCreateButton(ActionButtonContract $button): ActionButtonContract
{
return $button->error();
}
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyCreateButton(ActionButtonContract $button): ActionButtonContract
{
return $button->error();
}
You can also override the button.
use MoonShine\Contracts\UI\ActionButtonContract;
use MoonShine\UI\Components\ActionButton;
protected function modifyCreateButton(ActionButtonContract $button): ActionButtonContract
{
return ActionButton::make('Create');
}
namespaces
use MoonShine\Contracts\UI\ActionButtonContract;
use MoonShine\UI\Components\ActionButton;
protected function modifyCreateButton(ActionButtonContract $button): ActionButtonContract
{
return ActionButton::make('Create');
}
use MoonShine\Contracts\UI\ActionButtonContract;
use MoonShine\UI\Components\ActionButton;
protected function modifyCreateButton(ActionButtonContract $button): ActionButtonContract
{
return ActionButton::make('Create');
}
namespaces
use MoonShine\Contracts\UI\ActionButtonContract;
use MoonShine\UI\Components\ActionButton;
protected function modifyCreateButton(ActionButtonContract $button): ActionButtonContract
{
return ActionButton::make('Create');
}
use MoonShine\Contracts\UI\ActionButtonContract;
use MoonShine\UI\Components\ActionButton;
protected function modifyCreateButton(ActionButtonContract $button): ActionButtonContract
{
return ActionButton::make('Create');
}
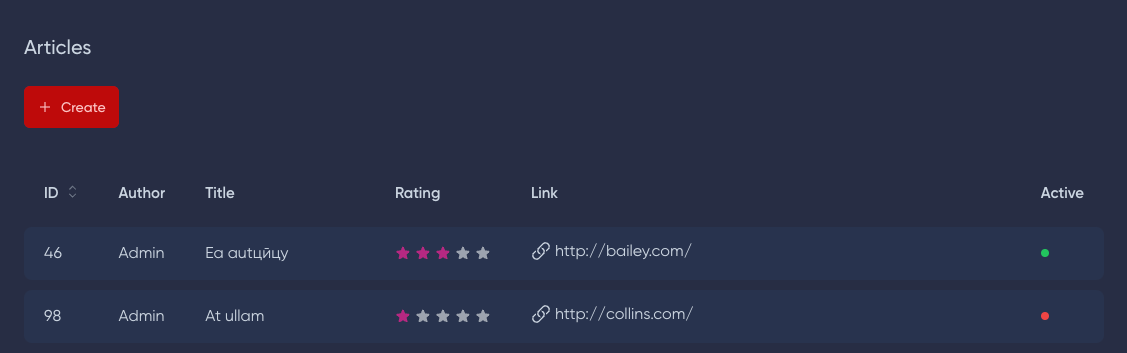
The modifyDetailButton()
method allows you to modify or override the button for viewing the details of an item.
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyDetailButton(ActionButtonContract $button): ActionButtonContract
{
return $button->warning();
}
namespaces
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyDetailButton(ActionButtonContract $button): ActionButtonContract
{
return $button->warning();
}
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyDetailButton(ActionButtonContract $button): ActionButtonContract
{
return $button->warning();
}
namespaces
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyDetailButton(ActionButtonContract $button): ActionButtonContract
{
return $button->warning();
}
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyDetailButton(ActionButtonContract $button): ActionButtonContract
{
return $button->warning();
}
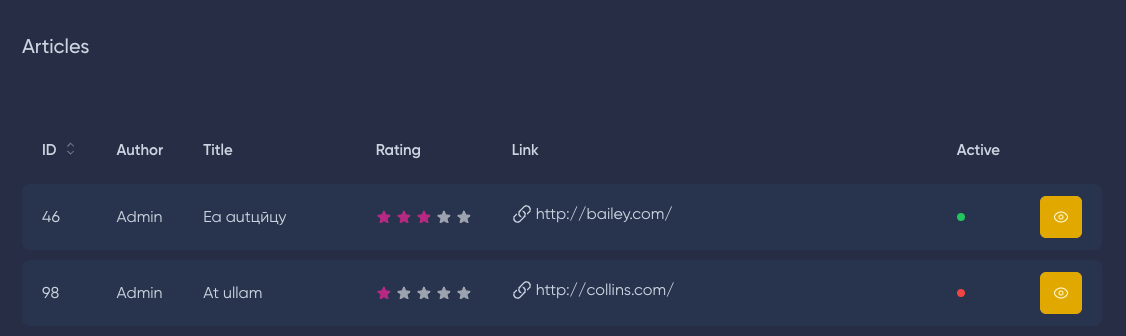
The modifyEditButton()
method allows you to modify or override the button for editing an item.
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyEditButton(ActionButtonContract $button): ActionButtonContract
{
return $button->icon('pencil-square');
}
namespaces
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyEditButton(ActionButtonContract $button): ActionButtonContract
{
return $button->icon('pencil-square');
}
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyEditButton(ActionButtonContract $button): ActionButtonContract
{
return $button->icon('pencil-square');
}
namespaces
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyEditButton(ActionButtonContract $button): ActionButtonContract
{
return $button->icon('pencil-square');
}
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyEditButton(ActionButtonContract $button): ActionButtonContract
{
return $button->icon('pencil-square');
}
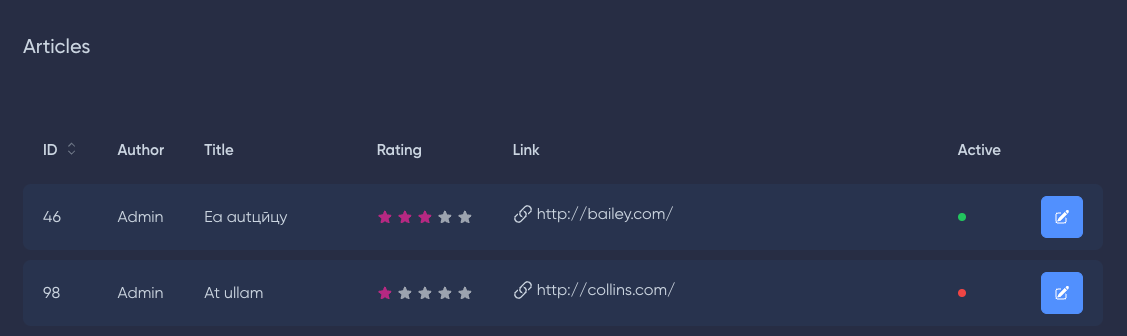
The modifyDeleteButton()
method allows you to modify or override the button for deleting an item.
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyDeleteButton(ActionButtonContract $button): ActionButtonContract
{
return $button->icon('x-mark');
}
namespaces
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyDeleteButton(ActionButtonContract $button): ActionButtonContract
{
return $button->icon('x-mark');
}
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyDeleteButton(ActionButtonContract $button): ActionButtonContract
{
return $button->icon('x-mark');
}
namespaces
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyDeleteButton(ActionButtonContract $button): ActionButtonContract
{
return $button->icon('x-mark');
}
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyDeleteButton(ActionButtonContract $button): ActionButtonContract
{
return $button->icon('x-mark');
}
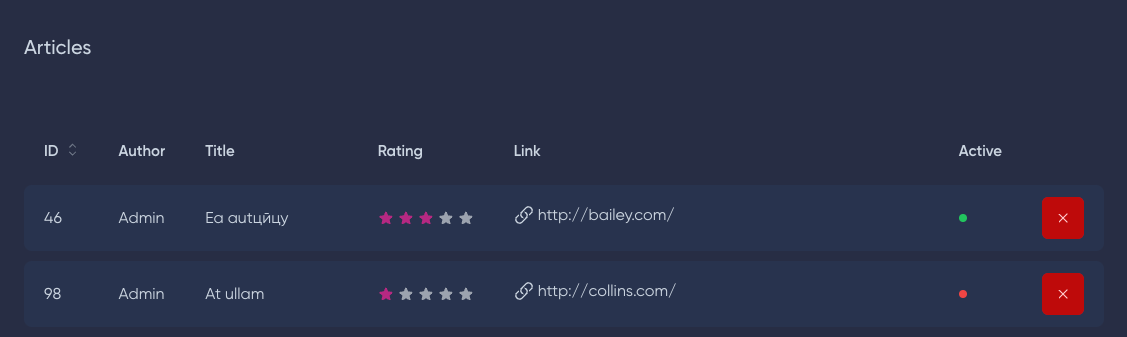
The modifyMassDeleteButton()
method allows you to modify or override the button for mass deleting.
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyMassDeleteButton(ActionButtonContract $button): ActionButtonContract
{
return $button->icon('x-mark');
}
namespaces
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyMassDeleteButton(ActionButtonContract $button): ActionButtonContract
{
return $button->icon('x-mark');
}
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyMassDeleteButton(ActionButtonContract $button): ActionButtonContract
{
return $button->icon('x-mark');
}
namespaces
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyMassDeleteButton(ActionButtonContract $button): ActionButtonContract
{
return $button->icon('x-mark');
}
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyMassDeleteButton(ActionButtonContract $button): ActionButtonContract
{
return $button->icon('x-mark');
}
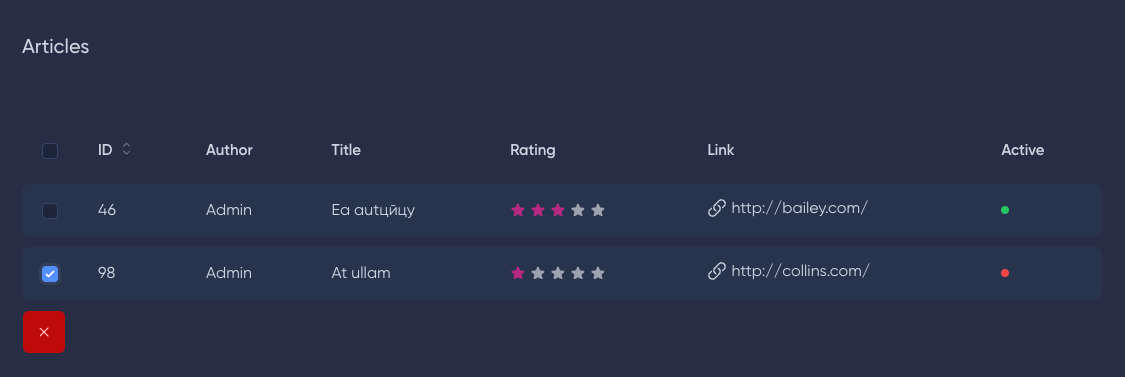
The modifyFiltersButton()
method allows you to modify or overwrite the filters button.
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyFiltersButton(ActionButtonContract $button): ActionButtonContract
{
return $button->error();
}
namespaces
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyFiltersButton(ActionButtonContract $button): ActionButtonContract
{
return $button->error();
}
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyFiltersButton(ActionButtonContract $button): ActionButtonContract
{
return $button->error();
}
namespaces
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyFiltersButton(ActionButtonContract $button): ActionButtonContract
{
return $button->error();
}
use MoonShine\Contracts\UI\ActionButtonContract;
protected function modifyFiltersButton(ActionButtonContract $button): ActionButtonContract
{
return $button->error();
}

By default, the model resource index page has only a create button.
The topButtons()
method allows you to add additional buttons.
use MoonShine\Support\AlpineJs;
use MoonShine\Support\Enums\JsEvent;
use MoonShine\Support\ListOf;
use MoonShine\UI\Components\ActionButton;
protected function topButtons(): ListOf
{
return parent::topButtons()
->add(
ActionButton::make('Refresh', '#')
->dispatchEvent(
AlpineJs::event(JsEvent::TABLE_UPDATED, $this->getListComponentName())
)
);
}
namespaces
use MoonShine\Support\AlpineJs;
use MoonShine\Support\Enums\JsEvent;
use MoonShine\Support\ListOf;
use MoonShine\UI\Components\ActionButton;
protected function topButtons(): ListOf
{
return parent::topButtons()
->add(
ActionButton::make('Refresh', '#')
->dispatchEvent(
AlpineJs::event(JsEvent::TABLE_UPDATED, $this->getListComponentName())
)
);
}
use MoonShine\Support\AlpineJs;
use MoonShine\Support\Enums\JsEvent;
use MoonShine\Support\ListOf;
use MoonShine\UI\Components\ActionButton;
protected function topButtons(): ListOf
{
return parent::topButtons()
->add(
ActionButton::make('Refresh', '#')
->dispatchEvent(
AlpineJs::event(JsEvent::TABLE_UPDATED, $this->getListComponentName())
)
);
}
namespaces
use MoonShine\Support\AlpineJs;
use MoonShine\Support\Enums\JsEvent;
use MoonShine\Support\ListOf;
use MoonShine\UI\Components\ActionButton;
protected function topButtons(): ListOf
{
return parent::topButtons()
->add(
ActionButton::make('Refresh', '#')
->dispatchEvent(
AlpineJs::event(JsEvent::TABLE_UPDATED, $this->getListComponentName())
)
);
}
use MoonShine\Support\AlpineJs;
use MoonShine\Support\Enums\JsEvent;
use MoonShine\Support\ListOf;
use MoonShine\UI\Components\ActionButton;
protected function topButtons(): ListOf
{
return parent::topButtons()
->add(
ActionButton::make('Refresh', '#')
->dispatchEvent(
AlpineJs::event(JsEvent::TABLE_UPDATED, $this->getListComponentName())
)
);
}
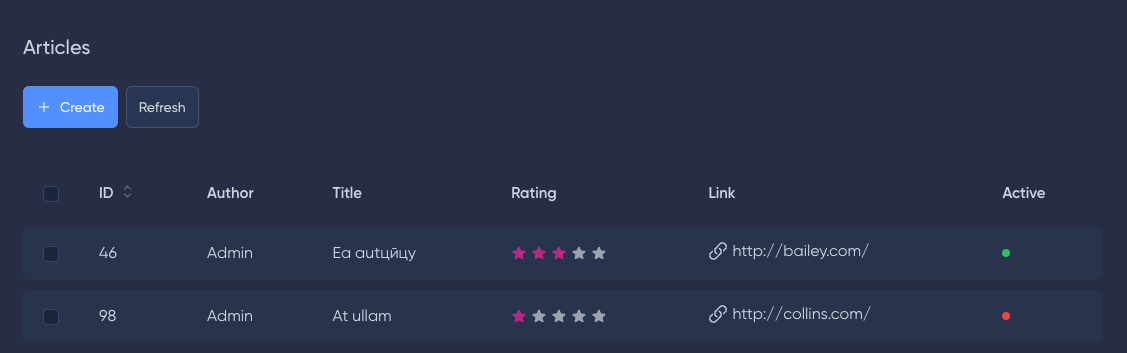
To add buttons in the index table, use the indexButtons()
method.
use Illuminate\Database\Eloquent\Model;
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function indexButtons(): ListOf
{
return parent::indexButtons()
->prepend(
ActionButton::make(
'Link',
fn(Model $item) => '/endpoint?id=' . $item->getKey()
)
);
}
namespaces
use Illuminate\Database\Eloquent\Model;
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function indexButtons(): ListOf
{
return parent::indexButtons()
->prepend(
ActionButton::make(
'Link',
fn(Model $item) => '/endpoint?id=' . $item->getKey()
)
);
}
use Illuminate\Database\Eloquent\Model;
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function indexButtons(): ListOf
{
return parent::indexButtons()
->prepend(
ActionButton::make(
'Link',
fn(Model $item) => '/endpoint?id=' . $item->getKey()
)
);
}
namespaces
use Illuminate\Database\Eloquent\Model;
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function indexButtons(): ListOf
{
return parent::indexButtons()
->prepend(
ActionButton::make(
'Link',
fn(Model $item) => '/endpoint?id=' . $item->getKey()
)
);
}
use Illuminate\Database\Eloquent\Model;
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function indexButtons(): ListOf
{
return parent::indexButtons()
->prepend(
ActionButton::make(
'Link',
fn(Model $item) => '/endpoint?id=' . $item->getKey()
)
);
}
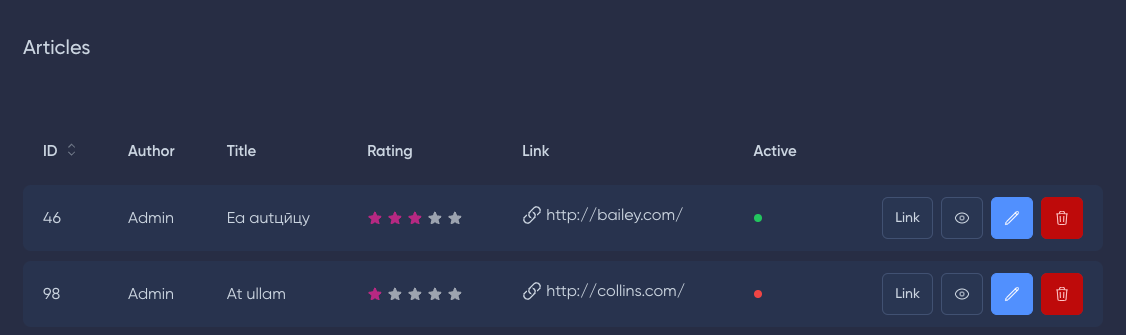
For bulk actions with elements, you need to add the bulk()
method.
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function indexButtons(): ListOf
{
return parent::indexButtons()
->prepend(
ActionButton::make('Link', '/endpoint')
->bulk()
);
}
namespaces
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function indexButtons(): ListOf
{
return parent::indexButtons()
->prepend(
ActionButton::make('Link', '/endpoint')
->bulk()
);
}
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function indexButtons(): ListOf
{
return parent::indexButtons()
->prepend(
ActionButton::make('Link', '/endpoint')
->bulk()
);
}
namespaces
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function indexButtons(): ListOf
{
return parent::indexButtons()
->prepend(
ActionButton::make('Link', '/endpoint')
->bulk()
);
}
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function indexButtons(): ListOf
{
return parent::indexButtons()
->prepend(
ActionButton::make('Link', '/endpoint')
->bulk()
);
}
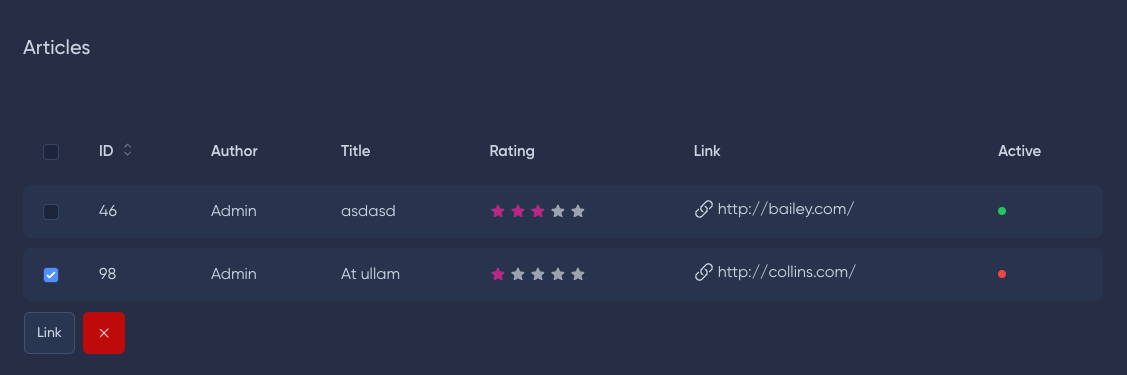
To add buttons to the form page, use the formButtons()
method.
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function formButtons(): ListOf
{
return parent::formButtons()
->add(
ActionButton::make('Link')->method('updateSomething')
);
}
namespaces
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function formButtons(): ListOf
{
return parent::formButtons()
->add(
ActionButton::make('Link')->method('updateSomething')
);
}
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function formButtons(): ListOf
{
return parent::formButtons()
->add(
ActionButton::make('Link')->method('updateSomething')
);
}
namespaces
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function formButtons(): ListOf
{
return parent::formButtons()
->add(
ActionButton::make('Link')->method('updateSomething')
);
}
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function formButtons(): ListOf
{
return parent::formButtons()
->add(
ActionButton::make('Link')->method('updateSomething')
);
}
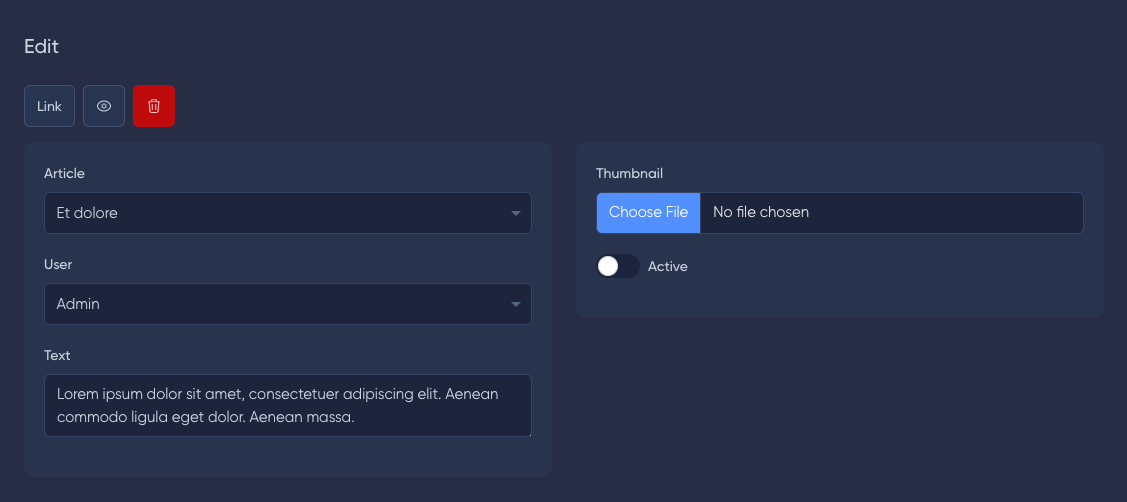
The formBuilderButtons()
method allows you to add additional buttons directly in the create or edit form.
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function formBuilderButtons(): ListOf
{
return parent::formBuilderButtons()
->add(
ActionButton::make('Back', fn() => $this->getIndexPageUrl())->class('btn-lg')
);
}
namespaces
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function formBuilderButtons(): ListOf
{
return parent::formBuilderButtons()
->add(
ActionButton::make('Back', fn() => $this->getIndexPageUrl())->class('btn-lg')
);
}
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function formBuilderButtons(): ListOf
{
return parent::formBuilderButtons()
->add(
ActionButton::make('Back', fn() => $this->getIndexPageUrl())->class('btn-lg')
);
}
namespaces
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function formBuilderButtons(): ListOf
{
return parent::formBuilderButtons()
->add(
ActionButton::make('Back', fn() => $this->getIndexPageUrl())->class('btn-lg')
);
}
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function formBuilderButtons(): ListOf
{
return parent::formBuilderButtons()
->add(
ActionButton::make('Back', fn() => $this->getIndexPageUrl())->class('btn-lg')
);
}
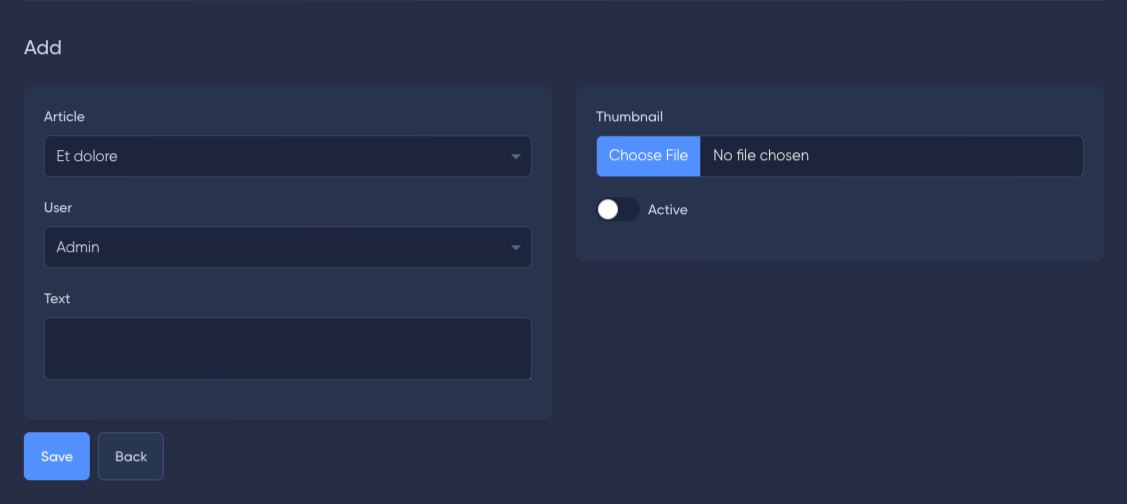
To add buttons to the detail view page, use the detailButtons()
method.
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function detailButtons(): ListOf
{
return parent::detailButtons()
->add(ActionButton::make('Link', '/endpoint'));
}
namespaces
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function detailButtons(): ListOf
{
return parent::detailButtons()
->add(ActionButton::make('Link', '/endpoint'));
}
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function detailButtons(): ListOf
{
return parent::detailButtons()
->add(ActionButton::make('Link', '/endpoint'));
}
namespaces
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function detailButtons(): ListOf
{
return parent::detailButtons()
->add(ActionButton::make('Link', '/endpoint'));
}
use MoonShine\UI\Components\ActionButton;
use MoonShine\Support\ListOf;
protected function detailButtons(): ListOf
{
return parent::detailButtons()
->add(ActionButton::make('Link', '/endpoint'));
}
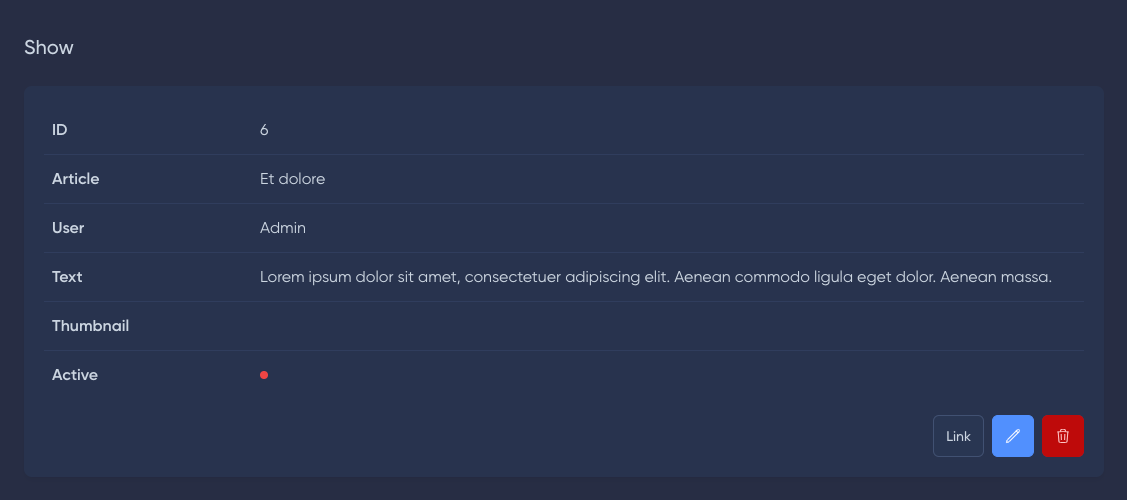